使用stm32F103c8t6编写打印串口的代码
时间: 2024-01-30 20:03:47 浏览: 44
以下是使用STM32F103C8T6编写打印串口的代码示例:
```c
#include "stm32f10x.h"
#include <stdio.h>
void USART1_Init(void);
void USART1_SendChar(char c);
void USART1_SendString(char* s);
int main(void)
{
USART1_Init();
USART1_SendString("Hello, World!\r\n");
while (1)
{
// main loop
}
}
void USART1_Init(void)
{
// Enable the USART1 peripheral clock
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);
// Configure the USART1 pins
GPIO_InitTypeDef GPIO_InitStruct;
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_9 | GPIO_Pin_10;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStruct);
// Configure the USART1 settings
USART_InitTypeDef USART_InitStruct;
USART_InitStruct.USART_BaudRate = 9600;
USART_InitStruct.USART_WordLength = USART_WordLength_8b;
USART_InitStruct.USART_StopBits = USART_StopBits_1;
USART_InitStruct.USART_Parity = USART_Parity_No;
USART_InitStruct.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStruct.USART_Mode = USART_Mode_Tx;
USART_Init(USART1, &USART_InitStruct);
// Enable the USART1 peripheral
USART_Cmd(USART1, ENABLE);
}
void USART1_SendChar(char c)
{
// Wait until the USART1 TX buffer is empty
while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
// Send the character
USART_SendData(USART1, c);
}
void USART1_SendString(char* s)
{
// Send each character in the string
while (*s)
{
USART1_SendChar(*s++);
}
}
```
在该示例中,我们使用USART1作为串口,并使用PA9和PA10引脚作为USART1的TX和RX引脚。我们将波特率设置为9600,并使用8位数据位,1位停止位和无校验位的配置。我们还提供了两个函数,`USART1_SendChar()`和`USART1_SendString()`,用于发送单个字符和字符串到串口。
在`main()`函数中,我们初始化了USART1并发送了一条“Hello, World!”消息到串口。在主循环中,我们可以添加其他操作。
相关推荐
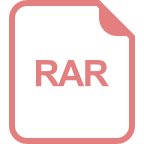
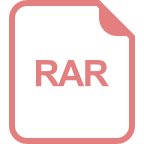
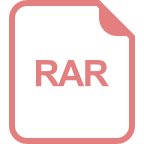
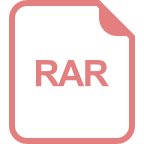
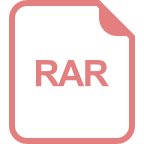
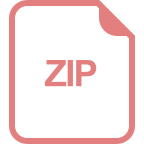



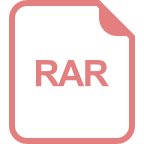
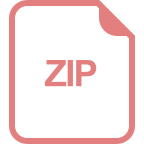
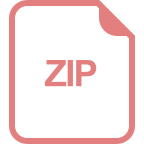
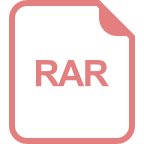
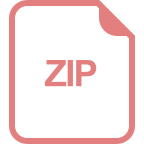
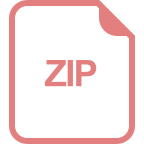
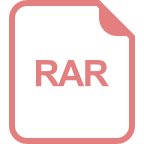