以二叉链表作为二叉树的存储结构,编写以下算法: 1.统计二叉树的叶结点个数。 2.判别两棵树是否相等。 3.交换二叉树每个结点的左孩子和右孩子。 4.设计二叉树的双序遍历算法
时间: 2024-01-23 16:46:24 浏览: 86
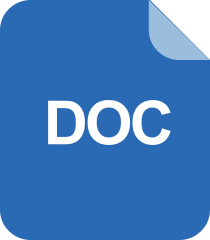
设二叉树采用链式存储结构,试设计一个算法计算一颗给定二叉树叶子节点的数目

1. 统计二叉树的叶结点个数
```python
def count_leaf_nodes(root):
if not root:
return 0
if not root.left and not root.right:
return 1
return count_leaf_nodes(root.left) + count_leaf_nodes(root.right)
```
2. 判别两棵树是否相等
```python
def is_same_tree(root1, root2):
if not root1 and not root2:
return True
if not root1 or not root2:
return False
if root1.val != root2.val:
return False
return is_same_tree(root1.left, root2.left) and is_same_tree(root1.right, root2.right)
```
3. 交换二叉树每个结点的左孩子和右孩子
```python
def swap_left_and_right(root):
if not root:
return
root.left, root.right = root.right, root.left
swap_left_and_right(root.left)
swap_left_and_right(root.right)
```
4. 设计二叉树的双序遍历算法
```python
def double_order_traversal(root):
if not root:
return
yield root.val
for val in double_order_traversal(root.left):
yield val
yield root.val
for val in double_order_traversal(root.right):
yield val
yield root.val
```
阅读全文
相关推荐









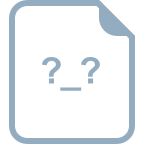
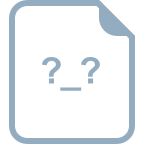
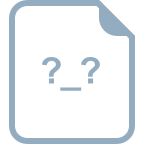
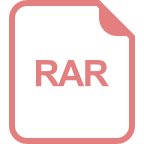