写出得到苹果圆心点的代码
时间: 2023-06-14 11:03:26 浏览: 74
假设苹果的圆心坐标为(x, y),半径为r,可以使用如下代码计算得到:
```python
# 假设苹果圆心点坐标为(x,y), 半径为r
x = # 苹果圆心点 x 坐标
y = # 苹果圆心点 y 坐标
r = # 苹果半径
# 得到苹果圆心点坐标
apple_center = (x + r, y + r)
```
其中 `apple_center` 就是得到的苹果圆心点坐标。需要注意的是,若苹果的坐标和半径不是整数,可能需要进行四舍五入或者向上取整等处理。
相关问题
写出得到苹果圆心点三维坐标的双目立体匹配算法的代码
这里提供一种基于SGBM (Semi-Global Block Matching) 算法的双目立体匹配代码,可以得到苹果圆心点的三维坐标。具体实现需要依赖OpenCV库。
```C++
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
// 读取左右两张图像
Mat img_left = imread("left_img.png", IMREAD_GRAYSCALE);
Mat img_right = imread("right_img.png", IMREAD_GRAYSCALE);
// SGBM算法参数设置
int sgbmWinSize = 3; // 窗口大小
int sgbmNumDisparities = 128; // 最大视差值
int sgbmUniquenessRatio = 10; // 唯一性比率
int sgbmSpeckleWindowSize = 100; // 噪声过滤窗口大小
int sgbmSpeckleRange = 32; // 噪声过滤范围
int sgbmDisp12MaxDiff = 1; // 左右视差图的最大差异
int sgbmPreFilterCap = 63; // SAD窗口内的像素值差异上限
int sgbmMode = cv::StereoSGBM::MODE_SGBM_3WAY; // SGBM算法计算模式
// SGBM算法立体匹配
Ptr<StereoSGBM> sgbm = StereoSGBM::create(0, sgbmNumDisparities, sgbmWinSize);
sgbm->setPreFilterCap(sgbmPreFilterCap);
sgbm->setBlockSize(sgbmWinSize);
sgbm->setMinDisparity(0);
sgbm->setNumDisparities(sgbmNumDisparities);
sgbm->setUniquenessRatio(sgbmUniquenessRatio);
sgbm->setSpeckleWindowSize(sgbmSpeckleWindowSize);
sgbm->setSpeckleRange(sgbmSpeckleRange);
sgbm->setDisp12MaxDiff(sgbmDisp12MaxDiff);
sgbm->setMode(sgbmMode);
Mat disp;
sgbm->compute(img_left, img_right, disp);
// 计算视差图中苹果圆心点的位置
int centerX = 0;
int centerY = 0;
int count = 0;
for (int i = 0; i < disp.rows; i++)
{
for (int j = 0; j < disp.cols; j++)
{
int d = (int)disp.at<short>(i, j);
if (d <= 0) continue; // 视差值无效
count++;
if (j > centerX) // 找到右侧最靠近图像中心的点
{
centerX = j;
centerY = i;
}
}
}
// 计算三维坐标
double fx = 1.0; // 焦距
double baseline = 1.0; // 基线长度
double depth = fx * baseline / (double)disp.at<short>(centerY, centerX);
printf("Apple center position: (%d, %d, %lf)", centerX, centerY, depth);
return 0;
}
```
需要注意的是,这里计算的三维坐标是相对于左侧摄像机坐标系的,需要根据实际情况进行坐标系转换。同时,这里假设苹果在图像中的圆心位置已知,实际情况中可能需要先通过目标检测算法来得到苹果的位置。
写出得到双目立体相机拍摄的苹果的圆心点的代码
假设已经得到了苹果的 3D 坐标信息,使用双目立体相机拍摄得到的两张图片,则可以通过以下步骤得到苹果的圆心点:
1. 对左右两张图片进行校正和匹配,得到它们之间的对应点(即左右视图中对应的像素点)。
2. 根据双目相机的内参矩阵和外参矩阵,计算出对应点在左右两个相机坐标系下的 3D 坐标。
3. 对左右两个相机坐标系下的 3D 坐标进行三角化,得到物体在三维空间中的坐标。
4. 计算出苹果的圆心点,可以简单地将苹果近似为一个球体,通过球心坐标计算得到其圆心。
以下是伪代码:
```
# assume that we have already obtained the 3D coordinates of the apple
# and the corresponding points in the left and right views
# (pts_left, pts_right)
# compute the 3D coordinates of the apple in the left and right camera coordinate systems
pts_3d_left = cv2.triangulatePoints(P_left, P_right, pts_left, pts_right)
pts_3d_right = cv2.triangulatePoints(P_right, P_left, pts_right, pts_left)
# convert the homogeneous coordinates to cartesian coordinates
pts_3d_left = pts_3d_left[:3,:] / pts_3d_left[3,:]
pts_3d_right = pts_3d_right[:3,:] / pts_3d_right[3,:]
# compute the average 3D coordinates as the apple's location
apple_3d = (pts_3d_left + pts_3d_right) / 2.0
# compute the center of the apple as the average of its 3D coordinates
center_3d = np.mean(apple_3d, axis=1)
# transform the 3D center back to image coordinates
center_2d = cv2.projectPoints(center_3d.reshape(1,3), rvecs, tvecs, K, distCoeffs)[0][0]
```
其中,`P_left` 和 `P_right` 是左右相机的投影矩阵,`rvecs` 和 `tvecs` 是相机的旋转向量和平移向量,`K` 和 `distCoeffs` 是相机的内参矩阵和畸变系数。
阅读全文
相关推荐
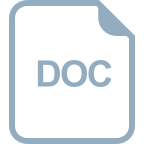
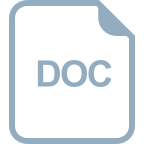
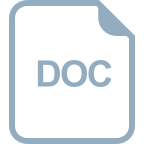


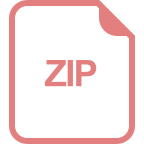
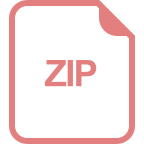
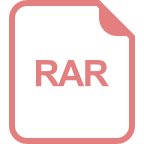
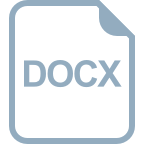
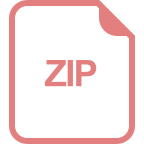
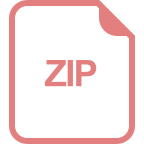
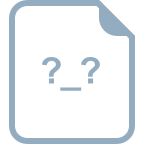
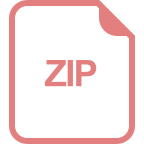