请写一段python代码,实现xml数据集格式转coco数据集json格式
时间: 2023-11-06 09:02:50 浏览: 116
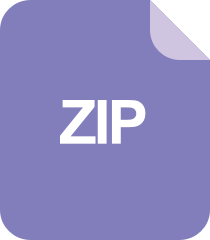
coco格式的json数据集读取保存为yolo的txt格式
以下是一个简单的Python程序,将XML格式的数据集转换为COCO格式的JSON数据集:
```python
import os
import json
import xml.etree.ElementTree as ET
# COCO 数据集的基本结构
coco_data = {
"images": [],
"annotations": [],
"categories": []
}
# 以下是你自己的类别和标注ID
classes_map = {
"car": 1,
"truck": 2,
"bus": 3
}
annotation_id = 1
# 以下是 XML 文件的路径和 COCO JSON 数据集输出路径
xml_path = "path/to/xml/files"
json_path = "path/to/coco/json"
# 遍历 XML 文件
for xml_file in os.listdir(xml_path):
if xml_file.endswith(".xml"):
# 解析 XML 文件
tree = ET.parse(os.path.join(xml_path, xml_file))
root = tree.getroot()
# 从 XML 文件中获取图像信息
image_id = int(root.find("filename").text.split(".")[0])
image_width = int(root.find("size/width").text)
image_height = int(root.find("size/height").text)
image_file_name = root.find("filename").text
# 添加图像信息到 COCO 数据集中
coco_data["images"].append({
"id": image_id,
"file_name": image_file_name,
"width": image_width,
"height": image_height
})
# 获取并添加标注信息到 COCO 数据集中
for obj in root.findall("object"):
class_name = obj.find("name").text
bbox = obj.find("bndbox")
x_min = int(bbox.find("xmin").text)
y_min = int(bbox.find("ymin").text)
x_max = int(bbox.find("xmax").text)
y_max = int(bbox.find("ymax").text)
coco_data["annotations"].append({
"id": annotation_id,
"image_id": image_id,
"category_id": classes_map[class_name],
"bbox": [x_min, y_min, x_max - x_min, y_max - y_min],
"area": (x_max - x_min) * (y_max - y_min),
"iscrowd": 0
})
annotation_id += 1
# 添加类别信息到 COCO 数据集中
for class_name, class_id in classes_map.items():
coco_data["categories"].append({
"id": class_id,
"name": class_name
})
# 将 COCO 数据集保存为 JSON 文件
with open(json_path, "w") as json_file:
json.dump(coco_data, json_file)
```
请注意,该程序仅仅是一个简单的示例,你需要根据你的数据集结构和类别信息进行相应的修改和调整。
阅读全文
相关推荐
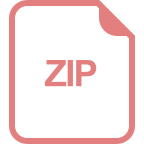
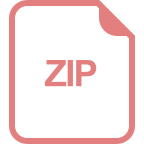















