商店销售某一商品,商店每天公布统一的折扣(discount),同时允许销售人员在销售时灵活的掌握售价(price),在此基础上,对一次购买10件以上者,还可以享受98折优惠,,请编写程序,计算出当日次商品的总销售额sum以及每件商品的平均售价,要求使用静态数据成员及静态成员函数。
时间: 2024-03-07 07:50:19 浏览: 145
以下是用C++实现的代码:
```c++
#include <iostream>
using namespace std;
class Goods {
private:
static float discount;
static int quantity_total;
static float price_total;
public:
int quantity;
float price;
static void set_discount(float d) {
discount = d;
}
static float get_discount() {
return discount;
}
static int get_quantity_total() {
return quantity_total;
}
static float get_price_total() {
return price_total;
}
Goods(int q, float p) {
quantity = q;
price = p;
quantity_total += q;
price_total += q * p;
}
float total_price() {
if (quantity >= 10) {
return quantity * price * discount;
} else {
return quantity * price;
}
}
static float average_price() {
return price_total / quantity_total;
}
};
float Goods::discount = 1;
int Goods::quantity_total = 0;
float Goods::price_total = 0;
int main() {
float discount;
cout << "请输入折扣:";
cin >> discount;
Goods::set_discount(discount);
int n;
cout << "请输入销售员数量:";
cin >> n;
Goods* goods[n];
float sum = 0;
for (int i = 0; i < n; i++) {
int quantity;
float price;
cout << "请输入第" << i + 1 << "个销售员的销售件数和销售单价:";
cin >> quantity >> price;
goods[i] = new Goods(quantity, price);
sum += goods[i]->total_price();
}
float average = Goods::average_price();
cout << "总销售额:" << sum << endl;
cout << "平均售价:" << average << endl;
return 0;
}
```
使用静态数据成员记录商品的总销售件数和总销售额,使用静态成员函数计算平均售价。通过构造函数初始化商品的数量和单价,计算出商品的总售价。在main函数中输入折扣和销售员数量,动态创建商品对象数组,计算总销售额和平均售价并输出即可。
阅读全文
相关推荐
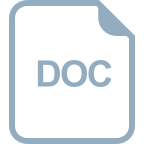
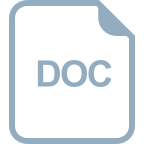
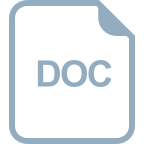















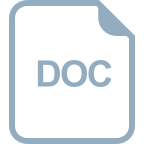