在qt 中的QLineF 在场景中传入两个坐标计算 两线段之间的夹角
时间: 2024-02-06 12:09:52 浏览: 249
可以通过QLineF类的`angleTo()`方法来计算两条线段之间的夹角。具体的实现步骤如下:
1. 创建两个QPointF对象,表示两个线段的起点和终点坐标;
2. 通过这两个QPointF对象创建两个QLineF对象;
3. 调用第一个QLineF对象的`angleTo()`方法,传入第二个QLineF对象,得到两条线段之间的夹角度数;
4. 将夹角度数转换为弧度。
下面是一个示例代码:
```cpp
QPointF p1(0, 0);
QPointF p2(100, 100);
QPointF p3(0, 0);
QPointF p4(100, 0);
QLineF line1(p1, p2);
QLineF line2(p3, p4);
qreal angle = line1.angleTo(line2);
qreal angleInRadian = qDegreesToRadians(angle);
```
在上面的代码中,`p1`和`p2`表示第一条线段的起点和终点,`p3`和`p4`表示第二条线段的起点和终点。`line1`和`line2`分别表示两条线段,`angle`表示两条线段之间的夹角度数,`angleInRadian`表示夹角的弧度值。
相关问题
qt中 在鼠标的按下的事件设置一条QLineF 并设置起始坐标和结束坐标, 鼠标移动事件也设置一条QLineF设置起始坐标和结束坐标并计算两线段之间夹角
可以通过以下代码实现:
```cpp
void MyWidget::mousePressEvent(QMouseEvent *event)
{
if (event->button() == Qt::LeftButton) {
startPoint = event->pos();
endPoint = startPoint;
}
}
void MyWidget::mouseMoveEvent(QMouseEvent *event)
{
if (event->buttons() & Qt::LeftButton) {
endPoint = event->pos();
update();
}
}
void MyWidget::paintEvent(QPaintEvent *event)
{
Q_UNUSED(event);
QPainter painter(this);
painter.setRenderHint(QPainter::Antialiasing);
// 绘制当前的线段
painter.drawLine(startPoint, endPoint);
// 计算两条线段的夹角
QPointF v1 = startPoint - endPoint;
QPointF v2 = lastPoint - currentPoint;
qreal angle = acos(QPointF::dotProduct(v1, v2) / (v1.manhattanLength() * v2.manhattanLength())) * 180 / M_PI;
// 绘制夹角
painter.setPen(Qt::red);
painter.drawText(endPoint.x(), endPoint.y(), QString::number(angle));
}
```
其中,`startPoint` 和 `endPoint` 分别表示当前绘制线段的起点和终点,`lastPoint` 和 `currentPoint` 分别表示前一次绘制线段的起点和终点。在 `mousePressEvent` 中记录当前点为起点,`mouseMoveEvent` 中更新终点,并在 `paintEvent` 中绘制当前的线段和计算两条线段的夹角并绘制出来。注意计算夹角时需要使用向量的点积和模长。
在鼠标的按下的事件设置一条QLineF 并设置起始坐标和结束坐标, 鼠标移动事件也设置一条QLineF设置起始坐标和结束坐标并计算两线段之间夹角
以下是一个示例代码,实现了在鼠标按下和移动时创建并更新一条线段,并计算两条线段之间的夹角:
```python
from PyQt5.QtCore import Qt, QPointF, QLineF, QPointF
from PyQt5.QtGui import QPainter, QPen
from PyQt5.QtWidgets import QApplication, QGraphicsScene, QGraphicsView, QGraphicsLineItem, QMainWindow
class MyView(QGraphicsView):
def __init__(self):
super().__init__()
self.scene = QGraphicsScene(self)
self.setScene(self.scene)
self.line1 = None
self.line2 = None
self.angle = None
def mousePressEvent(self, event):
if event.button() == Qt.LeftButton:
self.line1 = QLineF(event.scenePos(), event.scenePos())
self.line2 = None
self.angle = None
def mouseMoveEvent(self, event):
if self.line1 is not None:
self.line2 = QLineF(self.line1.p1(), event.scenePos())
if self.angle is not None:
self.angle = self.line1.angleTo(self.line2)
self.update()
def mouseReleaseEvent(self, event):
if event.button() == Qt.LeftButton:
if self.line2 is not None:
self.angle = self.line1.angleTo(self.line2)
self.scene.addItem(QGraphicsLineItem(self.line2))
self.line1 = None
self.line2 = None
self.update()
def paintEvent(self, event):
super().paintEvent(event)
if self.line1 is not None:
pen = QPen(Qt.red, 2, Qt.SolidLine, Qt.RoundCap)
self.scene.addLine(self.line1, pen)
if self.line2 is not None:
pen = QPen(Qt.green, 2, Qt.SolidLine, Qt.RoundCap)
self.scene.addLine(self.line2, pen)
if self.angle is not None:
painter = QPainter(self.viewport())
painter.setPen(QPen(Qt.black))
painter.drawText(QPointF(10, 20), "Angle: {:.2f}".format(self.angle))
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.view = MyView()
self.setCentralWidget(self.view)
if __name__ == '__main__':
app = QApplication([])
window = MainWindow()
window.show()
app.exec_()
```
在这个示例代码中,我们创建了一个自定义的 QGraphicsView 类,其中实现了三个鼠标事件:
- mousePressEvent:在左键按下时创建一条新的线段 line1,并将其起始点和结束点设置为当前鼠标位置。
- mouseMoveEvent:在左键按下并移动时,更新 line1 的结束点为当前鼠标位置,并创建一条新的线段 line2,将其起始点设置为 line1 的起始点,结束点设置为当前鼠标位置。同时计算 line1 和 line2 之间的夹角,并存储在 angle 变量中。
- mouseReleaseEvent:在左键释放时,如果当前存在 line2,则将其添加到场景中。同时清空 line1、line2 和 angle 变量。
在 paintEvent 方法中,我们根据当前的 line1、line2 和 angle 变量,分别在场景中绘制红色的 line1、绿色的 line2 和黑色的 angle 文本。由于我们只需要在视图中绘制这些元素,因此使用 viewport() 方法获取视图的绘制区域。
需要注意的是,在鼠标移动时计算夹角时,我们需要使用 QLineF 的 angleTo 方法,该方法可以计算两条线段之间的夹角。
阅读全文
相关推荐
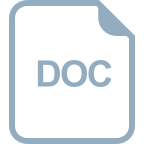












