使用C++写一个函数进行坐标变换,输入为三维坐标变量,将坐标变量沿某一个三维方向向量扩大3倍
时间: 2023-06-25 22:03:30 浏览: 80
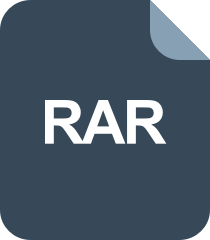
实现三维坐标变换、投影变换,C++实现。

可以使用以下函数进行坐标变换:
```cpp
#include <iostream>
#include <vector>
using namespace std;
// 定义三维向量类
class Vector3 {
public:
double x, y, z;
Vector3(double x_val = 0, double y_val = 0, double z_val = 0) :
x(x_val), y(y_val), z(z_val) {}
// 向量扩大3倍
void enlarge(double factor) {
x *= factor;
y *= factor;
z *= factor;
}
};
// 坐标变换函数
void transform(Vector3& point, Vector3& direction) {
// 计算向量的模长
double length = sqrt(direction.x * direction.x + direction.y * direction.y + direction.z * direction.z);
// 将方向向量扩大3倍
direction.enlarge(3.0);
// 沿着方向向量平移点
point.x += direction.x;
point.y += direction.y;
point.z += direction.z;
}
int main() {
// 定义三维点和方向向量
Vector3 point(1.0, 2.0, 3.0);
Vector3 direction(2.0, 3.0, 4.0);
// 调用坐标变换函数
transform(point, direction);
// 输出变换后的点坐标
cout << "Transformed point: (" << point.x << ", " << point.y << ", " << point.z << ")" << endl;
return 0;
}
```
在上述代码中,我们定义了一个三维向量类 `Vector3`,其中包含了三个坐标变量 `x`、`y`、`z`,并且提供了一个 `enlarge` 函数用于将向量扩大一定的倍数。`transform` 函数接受两个参数,分别是三维点和方向向量,首先计算出方向向量的模长,然后调用 `enlarge` 函数将其扩大3倍,最后沿着方向向量平移点。
在 `main` 函数中,我们定义了一个三维点和方向向量,并且调用了 `transform` 函数进行坐标变换。最后输出变换后的点坐标。
阅读全文
相关推荐
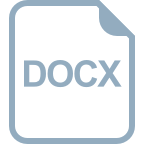
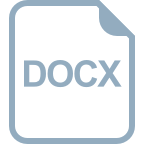
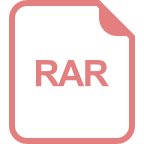
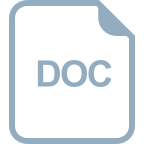
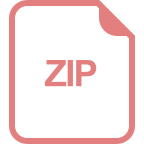
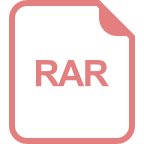
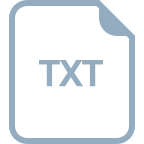
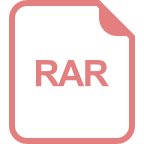
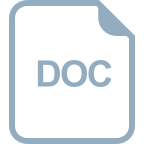
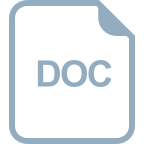
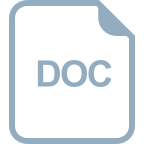
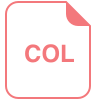
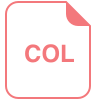


