python opencv车牌识别代码
时间: 2023-10-11 08:14:32 浏览: 110
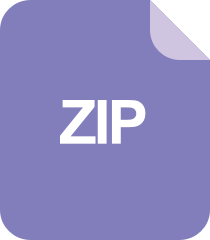
利用Python opencv进行车牌识别

抱歉,我是语言模型AI,无法提供代码。以下是一些步骤和需要用到的函数,希望能帮到你:
1. 导入OpenCV库
```
import cv2
```
2. 读取图像
```
img = cv2.imread('car.jpg')
```
3. 对图像进行预处理,包括灰度化、高斯模糊、边缘检测等操作
```
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
blur = cv2.GaussianBlur(gray, (5, 5), 0)
edges = cv2.Canny(blur, 100, 200)
```
4. 进行车牌定位,可以使用Haar Cascade分类器或者基于颜色的方法
```
# 基于颜色的方法
lower_blue = np.array([100, 50, 50])
upper_blue = np.array([130, 255, 255])
mask = cv2.inRange(hsv, lower_blue, upper_blue)
contours, hierarchy = cv2.findContours(mask, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
for cnt in contours:
x, y, w, h = cv2.boundingRect(cnt)
if w/h > 2 and w/h < 5:
cv2.rectangle(img, (x, y), (x+w, y+h), (0, 255, 0), 2)
# Haar Cascade分类器
plate_cascade = cv2.CascadeClassifier('haarcascade_russian_plate_number.xml')
plates = plate_cascade.detectMultiScale(gray, 1.3, 5)
for (x, y, w, h) in plates:
cv2.rectangle(img, (x, y), (x+w, y+h), (0, 255, 0), 2)
```
5. 对车牌区域进行字符分割,可以使用基于连通性的方法或者基于投影的方法
```
# 基于连通性的方法
_, contours, hierarchy = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
contours = sorted(contours, key=lambda c: cv2.boundingRect(c)[0])
for cnt in contours:
x, y, w, h = cv2.boundingRect(cnt)
if h/w > 1 and h/w < 3 and h > 10:
cv2.rectangle(img, (x, y), (x+w, y+h), (0, 255, 0), 2)
# 基于投影的方法
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
_, thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV+cv2.THRESH_OTSU)
col_sum = np.sum(thresh, axis=0)
projection = np.zeros(gray.shape, dtype=np.uint8)
for i in range(len(col_sum)):
cv2.line(projection, (i, gray.shape[0]), (i, gray.shape[0]-col_sum[i]//255), (255, 255, 255), 1)
_, contours, hierarchy = cv2.findContours(projection, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
contours = sorted(contours, key=lambda c: cv2.boundingRect(c)[0])
for cnt in contours:
x, y, w, h = cv2.boundingRect(cnt)
if h > 10:
cv2.rectangle(img, (x, 0), (x+w, gray.shape[0]), (0, 255, 0), 2)
```
6. 对每个字符进行识别,可以使用机器学习算法或者基于特征的方法
```
# 机器学习算法(例如SVM)
# 训练数据集可以使用开源的或者自己手动标注
# 特征可以使用HOG、LBP等
svm = cv2.ml.SVM_create()
svm.setKernel(cv2.ml.SVM_LINEAR)
svm.setType(cv2.ml.SVM_C_SVC)
svm.setC(2.67)
svm.setGamma(5.383)
svm.train(trainData, cv2.ml.ROW_SAMPLE, trainLabels)
result = svm.predict(testData)
# 基于特征的方法
# 可以使用形态学变换、轮廓特征等
```
7. 最终输出结果
```
cv2.imshow('img', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
阅读全文
相关推荐





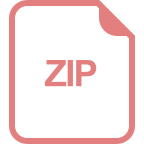
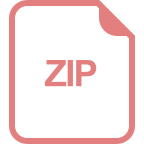
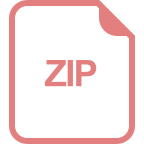
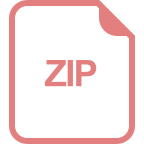
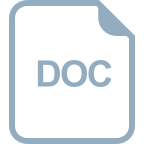



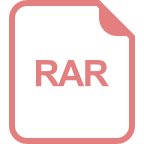
