c语言中怎么从键盘上读入学生信息:学号,姓名,年龄,成绩,根据成绩从高到低将学生信息输出
时间: 2023-06-04 18:07:22 浏览: 222
你可以使用 scanf 函数从键盘上读入学生信息,然后使用结构体存储学生信息,再使用排序算法(如冒泡排序、快速排序等)根据成绩从高到低将学生信息输出。代码实现详见以下示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 100
typedef struct {
char id[20];
char name[20];
int age;
float score;
} student;
int main() {
int n, i, j;
student stu[MAX_SIZE], temp;
printf("请输入学生人数:");
scanf("%d", &n);
for (i = 0; i < n; i++) {
printf("请输入第 %d 个学生的学号、姓名、年龄、成绩,用空格隔开:\n", i + 1);
scanf("%s %s %d %f", stu[i].id, stu[i].name, &stu[i].age, &stu[i].score);
}
// 冒泡排序
for (i = 0; i < n - 1; i++) {
for (j = 0; j < n - 1 - i; j++) {
if (stu[j].score < stu[j + 1].score) {
temp = stu[j];
stu[j] = stu[j + 1];
stu[j + 1] = temp;
}
}
}
// 输出学生信息
printf("学号\t\t姓名\t\t年龄\t\t成绩\n");
for (i = 0; i < n; i++) {
printf("%s\t%s\t%d\t%.2f\n", stu[i].id, stu[i].name, stu[i].age, stu[i].score);
}
return 0;
}
```
阅读全文
相关推荐
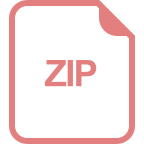
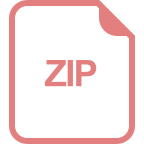
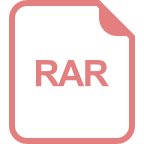















