device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
时间: 2023-09-15 08:20:25 浏览: 64
This line of code creates a device object using PyTorch library. It checks if a CUDA-enabled GPU is available and assigns the device as 'cuda' if it is, otherwise it assigns the device as 'cpu'. This helps to select the device for running the PyTorch computations. If a CUDA-enabled GPU is available, it is faster for running deep learning models than using the CPU.
相关问题
use_cuda = torch.cuda.is_available() FloatTensor = torch.cuda.FloatTensor if use_cuda else torch.FloatTensor device = torch.device("cpu") #使用GPU进行训练 from torch.autograd import Variable from replay_buffer import ReplayMemory, Transition # set up matplotlib is_ipython = 'inline' in matplotlib.get_backend() if is_ipython: from IPython import display #plt.ion() use_cuda = torch.cuda.is_available() FloatTensor = torch.cuda.FloatTensor if use_cuda else torch.FloatTensor device = torch.device("cpu")把这段代码改成CPU训练
您可以像这样将上述代码更改为在CPU上进行训练:
```
import torch
from torch.autograd import Variable
from replay_buffer import ReplayMemory, Transition
device = torch.device("cpu") # 使用CPU进行训练
# 设置matplotlib
is_ipython = 'inline' in matplotlib.get_backend()
if is_ipython:
from IPython import display
# 定义FloatTensor
FloatTensor = torch.FloatTensor
# 创建replay buffer
memory = ReplayMemory(10000)
# 将模型和优化器移动到CPU上
model = DQN().to(device)
optimizer = optim.Adam(model.parameters())
# 定义状态转换函数
def state_to_tensor(state):
return FloatTensor([state])
# 训练模型
for i in range(num_episodes):
state = env.reset()
for t in count():
# 获取动作
action = select_action(state)
# 执行动作并获取下一状态、奖励、是否结束
next_state, reward, done, _ = env.step(action.item())
# 转换状态和动作为张量
state_tensor = state_to_tensor(state)
action_tensor = LongTensor([action])
next_state_tensor = state_to_tensor(next_state)
# 存储状态转换
memory.push(state_tensor, action_tensor, next_state_tensor, reward)
# 更新状态
state = next_state
# 训练模型
optimize_model()
if done:
break
```
这里我们将使用CPU进行训练,所以我们将`device`设为`torch.device("cpu")`;将`FloatTensor`设为`torch.FloatTensor`即可。同时,我们还需要将模型和优化器移动到CPU上,可以使用`to`方法将它们移动到CPU上。
if torch.cuda.is_available(): torch.set_default_tensor_type(torch.cuda.FloatTensor) print("using cuda:", torch.cuda.get_device_name(0)) pass device = torch.device("cuda" if torch.cuda.is_available() else "cpu")这段代码是什么意思
这段代码的作用是,如果当前设备有可用的CUDA,则将默认的张量类型设置为CUDA浮点张量并输出使用的CUDA设备的名称。然后,它将设备设置为CUDA设备(如果有)或CPU。
具体来说:
- `torch.cuda.is_available()`:返回一个布尔值,表示当前设备是否有可用的CUDA。
- `torch.set_default_tensor_type(torch.cuda.FloatTensor)`:将默认的张量类型设置为CUDA浮点张量。
- `print("using cuda:", torch.cuda.get_device_name(0))`:输出使用的CUDA设备的名称。
- `device = torch.device("cuda" if torch.cuda.is_available() else "cpu")`:将设备设置为CUDA设备(如果有)或CPU。
阅读全文
相关推荐
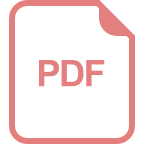
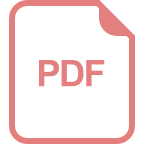
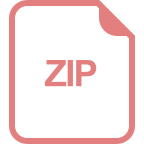











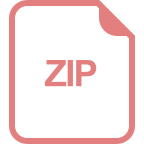
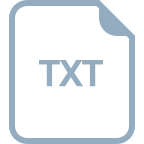
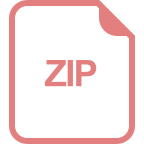