用指向对象的指针做函数参数,排序输出
时间: 2023-05-30 16:05:25 浏览: 146
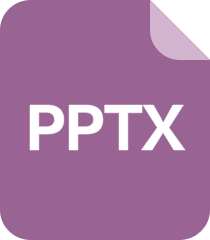
对象指针和对象引用作函数的参数-Read.pptx
以下是使用指向对象的指针作为函数参数,进行排序输出的示例代码:
```c++
#include <iostream>
#include <algorithm>
#include <string>
using namespace std;
class Person {
public:
string name;
int age;
Person(string name="", int age=0) {
this->name = name;
this->age = age;
}
bool operator<(const Person& other) const {
return age < other.age;
}
};
void print(const Person* arr[], int n) {
for (int i = 0; i < n; i++) {
cout << arr[i]->name << " " << arr[i]->age << endl;
}
}
void sortPersons(Person* arr[], int n) {
sort(arr, arr + n, [](const Person* a, const Person* b) {
return *a < *b;
});
}
int main() {
const int n = 4;
Person* persons[n];
persons[0] = new Person("Alice", 20);
persons[1] = new Person("Bob", 30);
persons[2] = new Person("Charlie", 25);
persons[3] = new Person("David", 18);
sortPersons(persons, n);
print(persons, n);
return 0;
}
```
这个程序定义了一个 `Person` 类,包含了一个姓名和一个年龄字段,并重载了 `<` 运算符,以便可以通过年龄来对 `Person` 对象进行排序。然后,定义了一个 `sortPersons` 函数,它使用 `std::sort` 函数对 `Person` 对象的指针进行排序。最后,定义了一个 `print` 函数,它可以输出指向 `Person` 对象的指针数组。在 `main` 函数中,创建了一个 `Person` 对象的指针数组,并对其进行排序和输出。
阅读全文
相关推荐
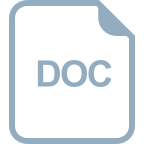
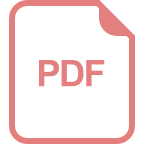
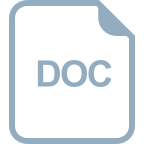
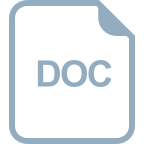
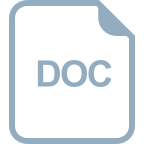
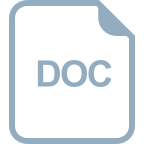
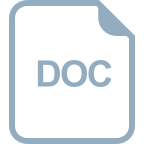








