6、输入某个点的平面坐标(x,y),判断该点在圆内、圆外还是在圆周上。其中圆心坐标为(2,2),半径为 1。c语言
时间: 2024-01-22 17:19:34 浏览: 137
以下是判断点是否在圆内、圆外还是在圆周上的 C 语言代码:
```c
#include <stdio.h>
#include <math.h>
int main() {
float x, y; // 输入点的坐标
float distance; // 点到圆心的距离
printf("请输入点的坐标(用空格隔开):");
scanf("%f %f", &x, &y);
// 计算点到圆心的距离
distance = sqrt(pow(x - 2, 2) + pow(y - 2, 2));
if (distance < 1) {
printf("该点在圆内\n");
} else if (distance > 1) {
printf("该点在圆外\n");
} else {
printf("该点在圆周上\n");
}
return 0;
}
```
运行程序后,输入点的坐标,程序会输出该点在圆内、圆外还是在圆周上。
相关问题
输入某个点的平面坐标(x,y),判断该点在圆内、圆外还是在圆周上。其中圆心坐标为(2,2),半径为1。 输入格式: 输入点的平面坐标,逗号分隔。
判断一个给定点是否在圆内、圆外或圆周上,可以使用距离公式计算该点到圆心的距离,然后与圆的半径进行比较。如果距离小于半径,则在圆内;等于半径则在圆周上;大于半径则在圆外。
假设输入的点的坐标为(x, y),圆心坐标为(2, 2),半径为1,首先计算两点之间的距离d:
\[ d = \sqrt{(x - 2)^2 + (y - 2)^2} \]
然后,我们有以下规则:
- 如果 \( d < 1 \),点在圆内;
- 如果 \( d = 1 \),点在圆周上;
- 如果 \( d > 1 \),点在圆外。
你可以通过编程实现这个算法,例如在Python中,你可以这样做:
```python
import math
def point_circle_position(x, y, center_x=2, center_y=2, radius=1):
distance = math.sqrt((x - center_x)**2 + (y - center_y)**2)
if distance < radius:
return "圆内"
elif distance == radius:
return "圆周上"
else:
return "圆外"
# 示例输入
input_point = input("请输入点的坐标,格式如(1, 1): ")
point = tuple(map(int, input_point[1:-1].split(',')))
print(point_circle_position(*point))
```
用户需要按照提示格式输入点的坐标,程序会返回相应的结果。
输入某个点的平面坐标(x,y),判断该点在圆内、圆外还是在圆周上。其中圆心坐标为(2,2),半 径为 1。
根据勾股定理,点 (x, y) 到圆心 (2, 2) 的距离为
$\sqrt{(x-2)^2+(y-2)^2}$
如果这个距离小于半径 1,则点在圆内;如果这个距离大于半径 1,则点在圆外;如果这个距离等于半径 1,则点在圆周上。
因此,可以写出如下的 Python 代码来判断点的位置:
```python
import math
def point_position(x, y):
distance = math.sqrt((x-2)**2 + (y-2)**2)
if distance < 1:
return "在圆内"
elif distance > 1:
return "在圆外"
else:
return "在圆周上"
# 测试
print(point_position(2, 2)) # 在圆周上
print(point_position(2, 3)) # 在圆外
print(point_position(3, 2)) # 在圆外
print(point_position(1, 2)) # 在圆内
```
其中,`math.sqrt()` 函数用于计算平方根。
阅读全文
相关推荐
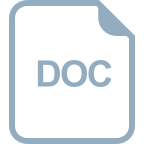
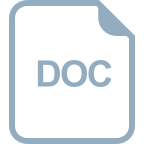
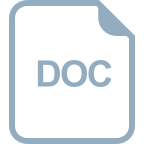






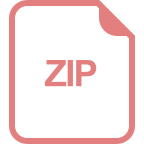
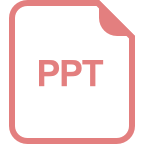
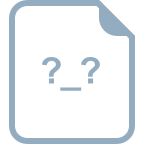
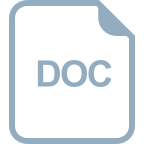
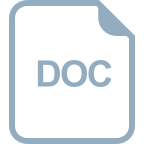


