用java创建一个学生类,从键盘上输入学生信息,可以把学生信息保存在指定文本文件中的指定位置;从文本文件中读取特定学生信息;实现学生信息的增加、删除、修改、插入等操作
时间: 2024-01-22 20:20:45 浏览: 122
以下是一个简单的Java学生类的实现,包括从键盘输入学生信息、将学生信息保存到指定文本文件中、从文本文件中读取特定学生信息、以及实现学生信息的增加、删除、修改、插入等操作。
```java
import java.io.*;
import java.util.*;
public class Student {
private String name;
private int age;
private String gender;
private String department;
private String id;
// 构造方法
public Student(String name, int age, String gender, String department, String id) {
this.name = name;
this.age = age;
this.gender = gender;
this.department = department;
this.id = id;
}
// 无参构造方法
public Student() {}
// toString方法
public String toString() {
return "姓名:" + name + ",年龄:" + age + ",性别:" + gender + ",所在系:" + department + ",学号:" + id;
}
// 从键盘输入学生信息
public static Student inputStudent() {
Scanner sc = new Scanner(System.in);
System.out.print("请输入学生姓名:");
String name = sc.nextLine();
System.out.print("请输入学生年龄:");
int age = sc.nextInt();
sc.nextLine();
System.out.print("请输入学生性别:");
String gender = sc.nextLine();
System.out.print("请输入学生所在系:");
String department = sc.nextLine();
System.out.print("请输入学生学号:");
String id = sc.nextLine();
return new Student(name, age, gender, department, id);
}
// 将学生信息保存到指定文本文件中
public static void saveStudentToFile(Student student, String filePath) {
try {
FileWriter fw = new FileWriter(filePath, true);
BufferedWriter bw = new BufferedWriter(fw);
bw.write(student.toString());
bw.newLine();
bw.close();
fw.close();
System.out.println("学生信息已保存到" + filePath + "文件中。");
} catch (IOException e) {
e.printStackTrace();
}
}
// 从文本文件中读取特定学生信息
public static List<Student> readStudentFromFile(String filePath, String id) {
List<Student> students = new ArrayList<>();
try {
FileReader fr = new FileReader(filePath);
BufferedReader br = new BufferedReader(fr);
String line;
while ((line = br.readLine()) != null) {
String[] fields = line.split(",");
if (fields[4].equals(id)) {
students.add(new Student(fields[0].substring(3), Integer.parseInt(fields[1].substring(4)), fields[2].substring(3), fields[3].substring(5), fields[4].substring(4)));
}
}
br.close();
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
return students;
}
// 实现学生信息的增加、删除、修改、插入等操作
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
List<Student> students = new ArrayList<>();
String filePath = "students.txt";
System.out.println("请选择操作:1.添加学生信息 2.删除学生信息 3.修改学生信息 4.插入学生信息 5.查询学生信息");
int choice = sc.nextInt();
switch (choice) {
case 1:
System.out.println("请输入要添加的学生信息:");
Student student = inputStudent();
students.add(student);
saveStudentToFile(student, filePath);
break;
case 2:
System.out.println("请输入要删除的学生学号:");
String deleteId = sc.next();
Iterator<Student> iter = students.iterator();
while (iter.hasNext()) {
Student s = iter.next();
if (s.id.equals(deleteId)) {
iter.remove();
break;
}
}
break;
case 3:
System.out.println("请输入要修改的学生学号:");
String modifyId = sc.next();
for (int i = 0; i < students.size(); i++) {
Student s = students.get(i);
if (s.id.equals(modifyId)) {
System.out.println("请输入修改后的学生信息:");
Student modifiedStudent = inputStudent();
students.set(i, modifiedStudent);
break;
}
}
break;
case 4:
System.out.println("请输入要插入的学生位置:");
int insertIndex = sc.nextInt();
System.out.println("请输入要插入的学生信息:");
Student insertedStudent = inputStudent();
students.add(insertIndex, insertedStudent);
break;
case 5:
System.out.println("请输入要查询的学生学号:");
String searchId = sc.next();
List<Student> searchedStudents = readStudentFromFile(filePath, searchId);
if (searchedStudents.size() == 0) {
System.out.println("未找到学号为" + searchId + "的学生信息。");
} else {
for (Student s : searchedStudents) {
System.out.println(s);
}
}
break;
default:
System.out.println("输入有误,请重新选择操作。");
break;
}
}
}
```
在上述代码中,我们通过一个简单的菜单来实现学生信息的增加、删除、修改、插入等操作。具体来说:
- 当用户选择1时,程序会从键盘输入学生信息,并将其保存到指定的文本文件中。
- 当用户选择2时,程序会从键盘输入要删除的学生学号,并在学生列表中查找该学生,如果找到则删除该学生信息。
- 当用户选择3时,程序会从键盘输入要修改的学生学号,并在学生列表中查找该学生,如果找到则从键盘输入修改后的学生信息,并将其更新到学生列表中。
- 当用户选择4时,程序会从键盘输入要插入的学生位置(索引),并从键盘输入要插入的学生信息,然后将其插入到学生列表中。
- 当用户选择5时,程序会从键盘输入要查询的学生学号,并从指定的文本文件中查找该学生信息,并输出到控制台。
需要注意的是,上述代码中的学生信息保存到文本文件中的格式为:
```
姓名:XXX,年龄:XX,性别:XXX,所在系:XXX,学号:XXXXX
```
因此,在从文本文件中读取学生信息时,我们需要按照这个格式进行解析。另外,为了方便起见,我们在程序中使用了Java集合类来管理学生列表,而不是使用数组等数据结构。
阅读全文
相关推荐






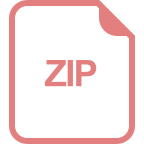

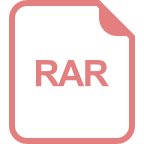
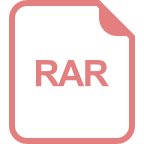






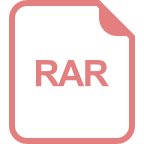
