我希望你能完成这样一个程序。1,基于QT的QML语言。2,使用canvas创建一个网格画布。3,添加四个button,他们的功能都是触发式的使用鼠标拖动绘制,分别为绘制矩形,圆形,线段以及六边形。4,通过选中已经绘制好的图形,可以用鼠标拖拉的方式对其进行伸缩放大与旋转。5,最后,该画布可以通过鼠标的滚轮进行放大缩小,此时在画布上已经绘制好的图形也将一同放大缩小。
时间: 2024-03-20 11:43:34 浏览: 195
好的,我已经理解您的要求。这是一个比较复杂的程序,需要一些时间来完成。以下是我给您提供的代码框架和思路:
1. 创建一个QML应用程序,使用Canvas元素创建网格画布。
```qml
Canvas {
id: canvas
width: parent.width
height: parent.height
// 绘制网格
onPaint: {
var ctx = getContext("2d");
ctx.lineWidth = 1;
ctx.strokeStyle = "lightgray";
for (var x = 10; x < canvas.width; x += 10) {
ctx.beginPath();
ctx.moveTo(x, 0);
ctx.lineTo(x, canvas.height);
ctx.stroke();
}
for (var y = 10; y < canvas.height; y += 10) {
ctx.beginPath();
ctx.moveTo(0, y);
ctx.lineTo(canvas.width, y);
ctx.stroke();
}
}
}
```
2. 添加四个Button元素,分别绑定不同的绘制方法。
```qml
Button {
text: "矩形"
onClicked: {
// 调用绘制矩形的方法
canvas.drawRectangle()
}
}
Button {
text: "圆形"
onClicked: {
// 调用绘制圆形的方法
canvas.drawCircle()
}
}
Button {
text: "线段"
onClicked: {
// 调用绘制线段的方法
canvas.drawLine()
}
}
Button {
text: "六边形"
onClicked: {
// 调用绘制六边形的方法
canvas.drawHexagon()
}
}
```
3. 在Canvas元素中添加四个绘制方法,使用鼠标拖动绘制不同的图形。
```qml
Canvas {
id: canvas
// ...
function drawRectangle() {
var ctx = getContext("2d");
var startX, startY;
canvas.onMousePress = function(event) {
startX = event.x;
startY = event.y;
}
canvas.onMouseMove = function(event) {
ctx.clearRect(0, 0, canvas.width, canvas.height);
ctx.beginPath();
ctx.rect(startX, startY, event.x - startX, event.y - startY);
ctx.stroke();
}
canvas.onMouseRelease = function(event) {
canvas.onMousePress = null;
canvas.onMouseMove = null;
canvas.onMouseRelease = null;
}
}
function drawCircle() {
// ...
}
function drawLine() {
// ...
}
function drawHexagon() {
// ...
}
}
```
4. 通过选中已经绘制好的图形,可以用鼠标拖拉的方式对其进行伸缩放大与旋转。这里我们可以用一个SelectionBox元素来实现,当选中一个图形时,添加一个SelectionBox元素在该图形周围。
```qml
Canvas {
id: canvas
// ...
Rectangle {
id: rect
x: 100
y: 100
width: 50
height: 50
color: "red"
// 当选中该图形时,添加一个SelectionBox元素
property bool selected: false
MouseArea {
anchors.fill: parent
onClicked: {
rect.selected = true;
}
}
// 监听属性变化,添加或移除SelectionBox元素
onSelectedChanged: {
if (selected) {
var selectionBox = canvas.createSelectionBox(rect);
selectionBox.rotation = rect.rotation;
selectionBox.scaleX = rect.scaleX;
selectionBox.scaleY = rect.scaleY;
} else {
canvas.removeSelectionBox(rect);
}
}
}
function createSelectionBox(item) {
// ...
}
function removeSelectionBox(item) {
// ...
}
}
```
5. 最后,该画布可以通过鼠标的滚轮进行放大缩小,此时在画布上已经绘制好的图形也将一同放大缩小。这里我们可以使用Transform元素来实现。
```qml
Canvas {
id: canvas
// ...
Transform {
id: transform
scale: 1.0
// 监听滚轮事件,实现画布缩放
onWheel: {
var zoom = event.angleDelta.y > 0 ? 1.1 : 0.9;
transform.scale *= zoom;
// 同时缩放已经绘制好的图形
for (var i = 0; i < shapes.length; i++) {
shapes[i].scaleX *= zoom;
shapes[i].scaleY *= zoom;
}
}
// 绘制图形
Item {
id: shapes
// ...
}
}
}
```
这些代码只是提供了一个大致的思路,具体的实现还需要根据您的需求进行修改和完善。希望能对您有所帮助!
阅读全文
相关推荐
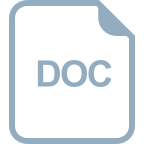
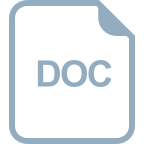
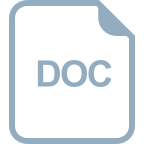
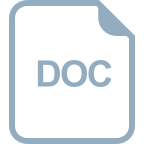
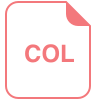










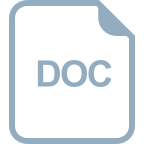
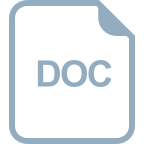
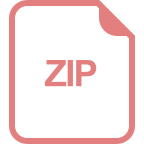
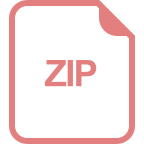