pygame实现俄罗斯方块
时间: 2023-11-12 10:14:38 浏览: 120
好的,下面我简单介绍一下如何使用pygame实现俄罗斯方块游戏。
首先,需要安装pygame模块。在命令行中输入以下命令即可安装:
```
pip install pygame
```
接下来,可以创建一个名为`tetris.py`的文件,并在该文件中编写游戏代码。
首先,导入pygame模块和一些常量:
```python
import pygame
from pygame.locals import *
import random
# 设置常量
SCREEN_WIDTH = 600
SCREEN_HEIGHT = 800
BLOCK_SIZE = 30
BLOCK_COLOR = (255, 255, 255) # 方块颜色
BG_COLOR = (0, 0, 0) # 背景颜色
```
然后,定义方块类和游戏类:
```python
# 方块类
class Block(pygame.sprite.Sprite):
def __init__(self, x, y):
super().__init__()
self.image = pygame.Surface((BLOCK_SIZE, BLOCK_SIZE))
self.image.fill(BLOCK_COLOR)
self.rect = self.image.get_rect()
self.rect.x = x
self.rect.y = y
def move_down(self):
self.rect.y += BLOCK_SIZE
def move_left(self):
self.rect.x -= BLOCK_SIZE
def move_right(self):
self.rect.x += BLOCK_SIZE
# 游戏类
class Tetris:
def __init__(self):
pygame.init()
self.screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption('Tetris')
self.clock = pygame.time.Clock()
self.score = 0
self.game_over = False
self.block_group = pygame.sprite.Group()
self.current_block = self.generate_block()
```
在游戏类中,我们通过`pygame.init()`初始化pygame,创建游戏窗口,并设置标题。然后,使用`pygame.time.Clock()`创建时钟对象,以便后面限制游戏帧率。我们还定义了一个分数变量和一个游戏结束状态变量。通过`pygame.sprite.Group()`创建方块精灵组,并使用`generate_block()`方法生成当前方块。
下面,我们编写一些方法来处理游戏逻辑:
```python
# 生成一个新方块
def generate_block(self):
block_type = random.randint(0, 6)
x = SCREEN_WIDTH // 2 - BLOCK_SIZE // 2
y = 0
if block_type == 0:
return IBlock(x, y)
elif block_type == 1:
return JBlock(x, y)
elif block_type == 2:
return LBlock(x, y)
elif block_type == 3:
return OBlock(x, y)
elif block_type == 4:
return SBlock(x, y)
elif block_type == 5:
return TBlock(x, y)
elif block_type == 6:
return ZBlock(x, y)
# 处理游戏事件
def handle_events(self):
for event in pygame.event.get():
if event.type == QUIT:
self.game_over = True
elif event.type == KEYDOWN:
if event.key == K_LEFT:
self.current_block.move_left()
elif event.key == K_RIGHT:
self.current_block.move_right()
elif event.key == K_DOWN:
self.current_block.move_down()
# 更新游戏状态
def update(self):
if len(pygame.sprite.spritecollide(self.current_block, self.block_group, False)) > 1:
self.current_block.rect.y -= BLOCK_SIZE
self.block_group.add(self.current_block)
self.current_block = self.generate_block()
self.current_block.move_down()
if self.current_block.rect.y > SCREEN_HEIGHT:
self.current_block.rect.y = 0
self.block_group.add(self.current_block)
self.current_block = self.generate_block()
for block in self.block_group:
if block.rect.y >= SCREEN_HEIGHT:
self.game_over = True
self.block_group.update()
# 绘制游戏界面
def draw(self):
self.screen.fill(BG_COLOR)
for block in self.block_group:
pygame.draw.rect(self.screen, block.image.get_at((0, 0)), block.rect)
pygame.display.update()
# 运行游戏
def run(self):
while not self.game_over:
self.handle_events()
self.update()
self.draw()
self.clock.tick(10)
pygame.quit()
```
`generate_block()`方法用于生成一个新的方块。我们随机生成一个方块类型,并根据类型生成相应的方块对象。
`handle_events()`方法用于处理游戏事件。我们通过`pygame.event.get()`获取所有事件,并根据事件类型来执行相应的操作。
`update()`方法用于更新游戏状态。我们首先判断当前方块是否与其它方块碰撞,如果是,则将当前方块添加到方块精灵组中,并生成一个新的方块。然后,让当前方块向下移动一格。如果当前方块已经到达底部,则将其添加到方块精灵组中,并生成一个新的方块。最后,遍历方块精灵组,如果有方块已经到达底部,则游戏结束。
`draw()`方法用于绘制游戏界面。我们首先填充背景颜色,然后遍历方块精灵组,绘制每个方块。
`run()`方法用于运行游戏。我们在一个循环中不断处理事件、更新状态和绘制界面,直到游戏结束为止。通过`clock.tick(10)`限制游戏帧率为10。
最后,我们定义各种不同类型的方块类,并在其中定义相应的移动方法:
```python
# I形方块
class IBlock(Block):
def __init__(self, x, y):
super().__init__(x, y)
self.image.fill((0, 255, 255))
def rotate(self):
pass
# J形方块
class JBlock(Block):
def __init__(self, x, y):
super().__init__(x, y)
self.image.fill((0, 0, 255))
def rotate(self):
pass
# L形方块
class LBlock(Block):
def __init__(self, x, y):
super().__init__(x, y)
self.image.fill((255, 165, 0))
def rotate(self):
pass
# O形方块
class OBlock(Block):
def __init__(self, x, y):
super().__init__(x, y)
self.image.fill((255, 255, 0))
def rotate(self):
pass
# S形方块
class SBlock(Block):
def __init__(self, x, y):
super().__init__(x, y)
self.image.fill((0, 255, 0))
def rotate(self):
pass
# T形方块
class TBlock(Block):
def __init__(self, x, y):
super().__init__(x, y)
self.image.fill((128, 0, 128))
def rotate(self):
pass
# Z形方块
class ZBlock(Block):
def __init__(self, x, y):
super().__init__(x, y)
self.image.fill((255, 0, 0))
def rotate(self):
pass
```
这些方块类都继承自`Block`类,并重写了`__init__()`方法以及`rotate()`方法(这里我们先不实现旋转功能)。
至此,我们就完成了俄罗斯方块游戏的实现。可以通过调用`Tetris().run()`来运行游戏。完整代码如下:
阅读全文
相关推荐
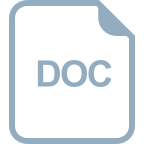
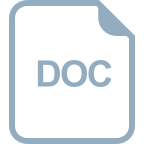
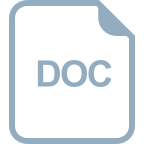
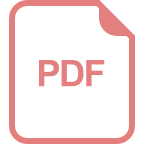
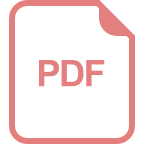
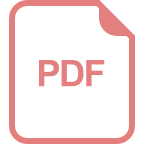
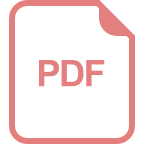
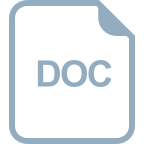
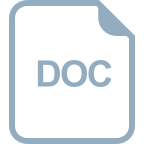
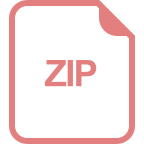
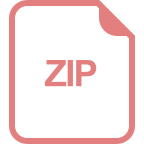
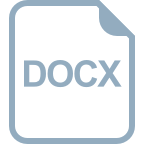
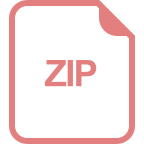