用c编写,程序开始时,自动从文件读入信息到结构体数组,并提取每个公民的出生年月放入结构体中,同时进行身份证校验位是否正确,判断信息也放入结构体中。用户选择1时,显示校验位正确的公民信息;用户选择1时,显示校验位正确的公民信息;用户选择2时,显示校验位错误的公民信息,并统计人数。用户选择2时,显示校验位错误的公民信息,并统计人数。用户选择3时,将正确公民信息按出生日期排序并输出。 用户选择4时,在正确公民信息中查找和指定姓名同名的人数。若用户选择0,会退出程序,但在此之前,会自动将身份证号码正确的数据写入文件person_checked.txt中。补全主函数,#include <stdio.h> #include <string.h> #define N 100 struct birth { int year; int month; int day; }; struct person { char ID[19]; char name[20]; struct birth birthday; int flag;//校验位是否正确, 1代表正确,0代表错误 }; int read(struct person p[]); int checkID(char *ID); struct birth get_birth(char *ID); void get_all_person(struct person p[], int n); void display_person(struct person p[], int n, int flag); int birth_cmp(struct birth birth1, struct birth birth2); void birth_sort(struct person p[], int n); int search(struct person p[], int n, char *name); void save(struct person p[], int n); int prompt(void); int main(void) { struct person p[N]; int cmd, n; while (1) { cmd = prompt(); } return 0; }
时间: 2023-06-25 13:04:28 浏览: 81
#include <stdio.h>
#include <string.h>
#define N 100
struct birth {
int year;
int month;
int day;
};
struct person {
char ID[19];
char name[20];
struct birth birthday;
int flag; //校验位是否正确,1代表正确,0代表错误
};
int read(struct person p[]);
int checkID(char *ID);
struct birth get_birth(char *ID);
void get_all_person(struct person p[], int n);
void display_person(struct person p[], int n, int flag);
int birth_cmp(struct birth birth1, struct birth birth2);
void birth_sort(struct person p[], int n);
int search(struct person p[], int n, char *name);
void save(struct person p[], int n);
int prompt(void);
int main(void) {
struct person p[N];
int cmd, n;
n = read(p); //从文件中读入信息到结构体数组
while (1) {
cmd = prompt();
switch (cmd) {
case 0:
save(p, n); //将身份证号码正确的数据写入文件person_checked.txt中
printf("程序已退出\n");
return 0;
case 1:
display_person(p, n, 1); //显示校验位正确的公民信息
break;
case 2:
display_person(p, n, 0); //显示校验位错误的公民信息,并统计人数
break;
case 3:
birth_sort(p, n); //将正确公民信息按出生日期排序并输出
break;
case 4:
printf("请输入要查找的姓名:");
char name[20];
scanf("%s", name);
int count = search(p, n, name); //在正确公民信息中查找和指定姓名同名的人数
printf("与%s同名的人数为%d\n", name, count);
break;
default:
printf("无效的命令,请重新输入\n");
}
}
return 0;
}
int read(struct person p[]) {
FILE *fp;
int n = 0;
fp = fopen("person.txt", "r");
if (fp == NULL) {
printf("文件读取失败\n");
return 0;
}
while (fscanf(fp, "%s%s", p[n].ID, p[n].name) != EOF) {
p[n].birthday = get_birth(p[n].ID);
p[n].flag = checkID(p[n].ID);
n++;
}
fclose(fp);
return n;
}
int checkID(char *ID) {
int weight[] = {7, 9, 10, 5, 8, 4, 2, 1, 6, 3, 7, 9, 10, 5, 8, 4, 2, 1};
char check_code[] = "10X98765432";
int sum = 0;
for (int i = 0; i < 17; i++) {
sum += (ID[i] - '0') * weight[i];
}
int remainder = sum % 11;
char code = check_code[remainder];
if (code == ID[17]) {
return 1;
} else {
return 0;
}
}
struct birth get_birth(char *ID) {
struct birth birth;
sscanf(ID + 6, "%4d%2d%2d", &birth.year, &birth.month, &birth.day);
return birth;
}
void get_all_person(struct person p[], int n) {
for (int i = 0; i < n; i++) {
printf("%s\t%s\t%d-%02d-%02d\t%s\n", p[i].ID, p[i].name, p[i].birthday.year, p[i].birthday.month, p[i].birthday.day, p[i].flag ? "正确" : "错误");
}
}
void display_person(struct person p[], int n, int flag) {
int count = 0;
for (int i = 0; i < n; i++) {
if (p[i].flag == flag) {
printf("%s\t%s\t%d-%02d-%02d\n", p[i].ID, p[i].name, p[i].birthday.year, p[i].birthday.month, p[i].birthday.day);
if (flag == 0) {
count++;
}
}
}
if (flag == 0) {
printf("共%d人\n", count);
}
}
int birth_cmp(struct birth birth1, struct birth birth2) {
if (birth1.year != birth2.year) {
return birth1.year - birth2.year;
} else if (birth1.month != birth2.month) {
return birth1.month - birth2.month;
} else {
return birth1.day - birth2.day;
}
}
void birth_sort(struct person p[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (birth_cmp(p[j].birthday, p[j+1].birthday) > 0) {
struct person temp = p[j];
p[j] = p[j+1];
p[j+1] = temp;
}
}
}
printf("身份证号\t姓名\t出生日期\t校验位\n");
get_all_person(p, n);
}
int search(struct person p[], int n, char *name) {
int count = 0;
for (int i = 0; i < n; i++) {
if (strcmp(p[i].name, name) == 0) {
count++;
}
}
return count;
}
void save(struct person p[], int n) {
FILE *fp;
fp = fopen("person_checked.txt", "w");
if (fp == NULL) {
printf("文件写入失败\n");
return;
}
for (int i = 0; i < n; i++) {
if (p[i].flag == 1) {
fprintf(fp, "%s\t%s\n", p[i].ID, p[i].name);
}
}
fclose(fp);
}
int prompt(void) {
int cmd;
printf("请选择功能:\n");
printf("1. 显示校验位正确的公民信息\n");
printf("2. 显示校验位错误的公民信息,并统计人数\n");
printf("3. 将正确公民信息按出生日期排序并输出\n");
printf("4. 在正确公民信息中查找和指定姓名同名的人数\n");
printf("0. 退出程序\n");
scanf("%d", &cmd);
return cmd;
}
阅读全文
相关推荐
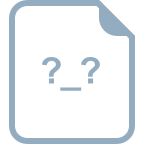
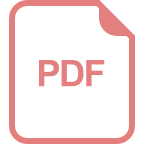
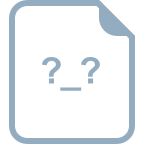














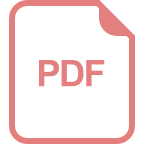
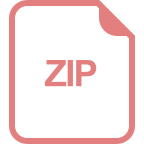