lr = LogisticRegression(verbose=1)
时间: 2024-06-07 20:11:25 浏览: 95
`lr = LogisticRegression(verbose=1)`是使用`LogisticRegression`类创建一个逻辑回归模型对象的代码。其中,`verbose=1`表示在训练过程中打印出损失函数值。
具体来说,`LogisticRegression`是一个用于解决二元分类问题的线性模型,它使用逻辑函数(也称为sigmoid函数)来估计概率。在这个例子中,我们使用`verbose=1`参数设置模型在训练过程中打印出损失函数值,以便我们能够监控模型的训练过程。
相关问题
lr = LogisticRegression()有什么参数可以设置
`LogisticRegression()`函数有很多参数可以设置,以下是一些常用的参数:
- `penalty`: 正则化项类型,默认为`l2`正则化
- `C`: 正则化强度的倒数,默认为1.0。较小的值表示更强的正则化
- `solver`: 优化算法类型,默认为`lbfgs`
- `max_iter`: 最大迭代次数,默认为100
- `multi_class`: 多分类问题的策略,默认为`auto`,表示根据数据自动选择算法。其他可选项有`ovr`和`multinomial`
- `class_weight`: 类别权重,可选项有`balanced`和自定义权重
- `verbose`: 是否输出详细信息,默认为0,表示不输出
具体参数及其含义可以参考`LogisticRegression`的官方文档。
把这段代码的PCA换成LDA:LR_grid = LogisticRegression(max_iter=1000, random_state=42) LR_grid_search = GridSearchCV(LR_grid, param_grid=param_grid, cv=cvx ,scoring=scoring,n_jobs=10,verbose=0) LR_grid_search.fit(pca_X_train, train_y) estimators = [ ('lr', LR_grid_search.best_estimator_), ('svc', svc_grid_search.best_estimator_), ] clf = StackingClassifier(estimators=estimators, final_estimator=LinearSVC(C=5, random_state=42),n_jobs=10,verbose=1) clf.fit(pca_X_train, train_y) estimators = [ ('lr', LR_grid_search.best_estimator_), ('svc', svc_grid_search.best_estimator_), ] param_grid = {'final_estimator':[LogisticRegression(C=0.00001),LogisticRegression(C=0.0001), LogisticRegression(C=0.001),LogisticRegression(C=0.01), LogisticRegression(C=0.1),LogisticRegression(C=1), LogisticRegression(C=10),LogisticRegression(C=100), LogisticRegression(C=1000)]} Stacking_grid =StackingClassifier(estimators=estimators,) Stacking_grid_search = GridSearchCV(Stacking_grid, param_grid=param_grid, cv=cvx, scoring=scoring,n_jobs=10,verbose=0) Stacking_grid_search.fit(pca_X_train, train_y) Stacking_grid_search.best_estimator_ train_pre_y = cross_val_predict(Stacking_grid_search.best_estimator_, pca_X_train,train_y, cv=cvx) train_res1=get_measures_gridloo(train_y,train_pre_y) test_pre_y = Stacking_grid_search.predict(pca_X_test) test_res1=get_measures_gridloo(test_y,test_pre_y) best_pca_train_aucs.append(train_res1.loc[:,"AUC"]) best_pca_test_aucs.append(test_res1.loc[:,"AUC"]) best_pca_train_scores.append(train_res1) best_pca_test_scores.append(test_res1) train_aucs.append(np.max(best_pca_train_aucs)) test_aucs.append(best_pca_test_aucs[np.argmax(best_pca_train_aucs)].item()) train_scores.append(best_pca_train_scores[np.argmax(best_pca_train_aucs)]) test_scores.append(best_pca_test_scores[np.argmax(best_pca_train_aucs)]) pca_comp.append(n_components[np.argmax(best_pca_train_aucs)]) print("n_components:") print(n_components[np.argmax(best_pca_train_aucs)])
如果要将代码中的PCA替换为LDA,可以按照以下步骤进行修改:
```python
LR_grid = LogisticRegression(max_iter=1000, random_state=42)
LR_grid_search = GridSearchCV(LR_grid, param_grid=param_grid, cv=cvx ,scoring=scoring,n_jobs=10,verbose=0)
LR_grid_search.fit(lda_X_train, train_y)
estimators = [
('lr', LR_grid_search.best_estimator_),
('svc', svc_grid_search.best_estimator_),
]
clf = StackingClassifier(estimators=estimators, final_estimator=LinearSVC(C=5, random_state=42),n_jobs=10,verbose=1)
clf.fit(lda_X_train, train_y)
estimators = [
('lr', LR_grid_search.best_estimator_),
('svc', svc_grid_search.best_estimator_),
]
param_grid = {'final_estimator': [LogisticRegression(C=0.00001),LogisticRegression(C=0.0001),
LogisticRegression(C=0.001),LogisticRegression(C=0.01),
LogisticRegression(C=0.1),LogisticRegression(C=1),
LogisticRegression(C=10),LogisticRegression(C=100),
LogisticRegression(C=1000)]}
Stacking_grid = StackingClassifier(estimators=estimators,)
Stacking_grid_search = GridSearchCV(Stacking_grid, param_grid=param_grid, cv=cvx,
scoring=scoring, n_jobs=10, verbose=0)
Stacking_grid_search.fit(lda_X_train, train_y)
Stacking_grid_search.best_estimator_
train_pre_y = cross_val_predict(Stacking_grid_search.best_estimator_, lda_X_train, train_y, cv=cvx)
train_res1 = get_measures_gridloo(train_y, train_pre_y)
test_pre_y = Stacking_grid_search.predict(lda_X_test)
test_res1 = get_measures_gridloo(test_y, test_pre_y)
best_lda_train_aucs.append(train_res1.loc[:,"AUC"])
best_lda_test_aucs.append(test_res1.loc[:,"AUC"])
best_lda_train_scores.append(train_res1)
best_lda_test_scores.append(test_res1)
train_aucs.append(np.max(best_lda_train_aucs))
test_aucs.append(best_lda_test_aucs[np.argmax(best_lda_train_aucs)].item())
train_scores.append(best_lda_train_scores[np.argmax(best_lda_train_aucs)])
test_scores.append(best_lda_test_scores[np.argmax(best_lda_train_aucs)])
lda_comp.append(n_components[np.argmax(best_lda_train_aucs)])
print("n_components:")
print(n_components[np.argmax(best_lda_train_aucs)])
```
在这个修改后的代码中,将`pca_X_train`和`pca_X_test`替换为`lda_X_train`和`lda_X_test`,并相应地修改变量和参数的名称。这样就可以使用LDA进行特征降维和模型训练了。
阅读全文
相关推荐
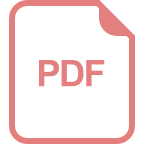
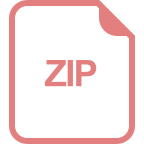



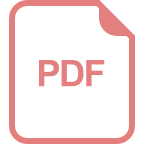








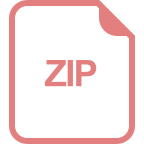