用c语言写一个将链表中数据排序的代码,链表中有首字母来排序姓名,用快速排序法将链表结点进行排序
时间: 2024-03-13 10:17:29 浏览: 67
好的,以下是用 C 语言实现链表排序的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义链表结构体
struct ListNode {
char name[20]; // 姓名
struct ListNode* next;
};
// 快速排序函数
void quickSort(struct ListNode* left, struct ListNode* right) {
if (left == NULL || right == NULL || left == right) {
return;
}
struct ListNode* p = left;
struct ListNode* q = p->next;
char tmp[20];
while (q != right->next) {
if (strcmp(q->name, left->name) < 0) {
p = p->next;
strcpy(tmp, p->name);
strcpy(p->name, q->name);
strcpy(q->name, tmp);
}
q = q->next;
}
strcpy(tmp, left->name);
strcpy(left->name, p->name);
strcpy(p->name, tmp);
quickSort(left, p);
quickSort(p->next, right);
}
int main() {
// 创建链表
struct ListNode* head = NULL;
struct ListNode* tail = NULL;
struct ListNode* pNode;
char name[20];
printf("请输入姓名(输入 end 结束):\n");
while (1) {
scanf("%s", name);
if (strcmp(name, "end") == 0) {
break;
}
pNode = (struct ListNode*)malloc(sizeof(struct ListNode));
strcpy(pNode->name, name);
pNode->next = NULL;
if (head == NULL) {
head = pNode;
tail = pNode;
} else {
tail->next = pNode;
tail = pNode;
}
}
// 对链表进行排序
quickSort(head, tail);
// 打印排序后的链表
printf("排序后的链表:\n");
pNode = head;
while (pNode != NULL) {
printf("%s\n", pNode->name);
pNode = pNode->next;
}
// 释放链表内存
pNode = head;
while (pNode != NULL) {
head = head->next;
free(pNode);
pNode = head;
}
return 0;
}
```
希望能帮到您!
阅读全文
相关推荐
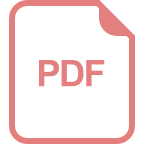














