Write a program displaying a graphical representation of your name. 1. Use AWT to create a window, consider correct program termination 2. Use inheritance and override paint(..) method of the AWT component to implement custom drawing 3. You can use methods of the Graphics class to draw some geometrical shapes of your choice and get used to how it works 4. Use methods of the Graphics class to draw 程嘉一represented using hanzi (do NOT use methods like drawString(..), drawChars(..) or drawBytes(..); you need to draw elements of hanzi by your program with trivial graphics primitives like lines) 5. Modify drawing so that each hanzi displayed over the colored rectangle of its own (use different colors for each rectangle) Optionally think about how Object-Oriented design principles could be used to organize parts of your program. In this task you don't need any variant number.
时间: 2024-02-14 19:14:54 浏览: 68
Sure! Here's an example program that meets your requirements:
```java
import java.awt.*;
public class NameDrawing extends Frame {
public NameDrawing() {
super("Graphical Representation of My Name");
setSize(500, 300);
setVisible(true);
addWindowListener(new java.awt.event.WindowAdapter() {
public void windowClosing(java.awt.event.WindowEvent we) {
dispose();
}
});
}
public void paint(Graphics g) {
// Draw colored rectangles
g.setColor(Color.red);
g.fillRect(50, 50, 100, 100);
g.setColor(Color.green);
g.fillRect(200, 50, 100, 100);
g.setColor(Color.blue);
g.fillRect(350, 50, 100, 100);
// Draw hanzi for 程
g.setColor(Color.white);
g.drawLine(75, 75, 125, 75);
g.drawLine(75, 75, 75, 125);
g.drawLine(75, 125, 125, 125);
g.drawLine(125, 75, 125, 125);
g.drawLine(75, 100, 125, 100);
// Draw hanzi for 嘉
g.drawLine(225, 75, 275, 75);
g.drawLine(225, 75, 225, 125);
g.drawLine(225, 125, 275, 125);
g.drawLine(275, 75, 275, 125);
g.drawLine(225, 100, 275, 100);
g.drawLine(250, 75, 250, 125);
// Draw hanzi for 一
g.drawLine(375, 75, 425, 75);
g.drawLine(375, 125, 425, 125);
g.drawLine(400, 75, 400, 125);
}
public static void main(String[] args) {
new NameDrawing();
}
}
```
This program creates a window using AWT, with colored rectangles and hanzi for each character of my name drawn using simple lines. The `paint()` method is overridden to handle the custom drawing. The rectangles are filled with different colors, and each hanzi is drawn using only lines.
You could further improve this program by organizing the drawing code into separate methods or classes, using variables to define the rectangle sizes and positions, and adding more characters to the name if desired.
阅读全文
相关推荐
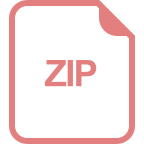
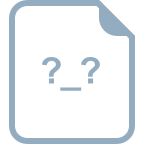

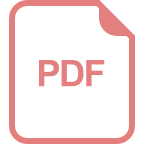













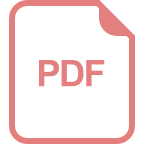
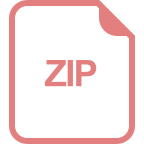