C语言判断arp协议是免费arp的代码
时间: 2023-12-08 10:04:04 浏览: 83
以下是使用C语言判断ARP协议是免费ARP的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <net/if.h>
#include <netinet/if_ether.h>
int is_free_arp(struct ether_arp *arp_packet) {
if (ntohs(arp_packet->arp_op) != ARPOP_REQUEST) { // 只处理ARP请求
return 0;
}
if (memcmp(arp_packet->arp_tha, "\x00\x00\x00\x00\x00\x00", 6) != 0) { // 目标MAC地址不是广播地址
return 0;
}
if (memcmp(arp_packet->arp_tpa, arp_packet->arp_spa, 4) != 0) { // 目标IP地址不是本地IP地址
return 0;
}
return 1;
}
int main(int argc, char *argv[]) {
int sockfd;
struct sockaddr_ll saddr;
struct ether_arp arp_packet;
unsigned char buffer[ETH_FRAME_LEN];
ssize_t n;
// 创建原始套接字
sockfd = socket(AF_PACKET, SOCK_RAW, htons(ETH_P_ARP));
if (sockfd < 0) {
perror("socket");
exit(EXIT_FAILURE);
}
// 绑定网络接口
memset(&saddr, 0, sizeof(saddr));
saddr.sll_family = AF_PACKET;
saddr.sll_protocol = htons(ETH_P_ARP);
saddr.sll_ifindex = if_nametoindex("eth0"); // 替换为网络接口名称
if (bind(sockfd, (struct sockaddr *)&saddr, sizeof(saddr)) < 0) {
perror("bind");
exit(EXIT_FAILURE);
}
// 接收并处理ARP请求
while (1) {
n = recv(sockfd, buffer, sizeof(buffer), 0);
if (n < 0) {
perror("recv");
exit(EXIT_FAILURE);
}
memcpy(&arp_packet, buffer + sizeof(struct ether_header), sizeof(struct ether_arp));
if (is_free_arp(&arp_packet)) {
printf("Received a free ARP request from %s\n", inet_ntoa(*(struct in_addr *)arp_packet.arp_spa));
}
}
close(sockfd);
return 0;
}
```
该代码使用了Linux下的原始套接字接收ARP请求,并判断是否为免费ARP请求。其中,`is_free_arp`函数用于判断一个ARP请求是否为免费ARP请求。如果是,则打印源IP地址。需要注意的是,运行该程序需要root权限。
阅读全文
相关推荐
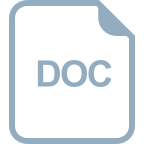
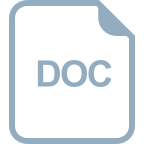
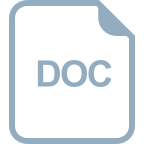

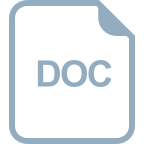
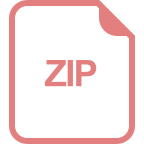
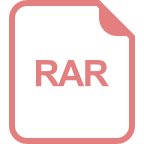
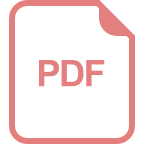
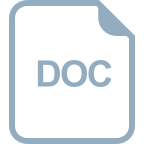
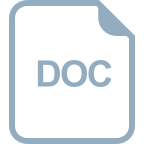
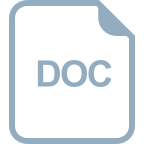



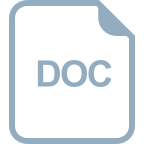
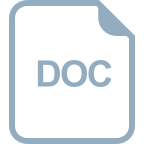

