<input type="button" @click="findAllProduct({{current-1}},4)" value="上页"/> <input type="button" @click="findAllProduct({{current+1}},4)" value="下页"/> <input type="button" @click="findAllProduct('+{{pages}}+',4)" value="末页"/>
时间: 2023-07-21 16:02:57 浏览: 40
非常抱歉,我之前的回答有误。在Vue.js中,我们不需要使用双花括号`{{}}`来插入变量,而是直接在指令中使用变量名称。
以下是修正后的代码示例:
```html
<input type="button" @click="findAllProduct(current - 1, 4)" value="上页"/>
<input type="button" @click="findAllProduct(current + 1, 4)" value="下页"/>
<input type="button" @click="findAllProduct(pages, 4)" value="末页"/>
```
修正后的代码中,使用了`@click`指令来监听按钮的点击事件,并直接传递变量给`findAllProduct`方法。
请确保在Vue实例中定义了`current`和`pages`这两个变量,并正确实现了`findAllProduct`方法来获取相应页码的产品数据。
相关问题
<input type="button" v-on:click="findAllProduct({{current-1}},4)" value="上页"/> <input type="button" v-on:click="findAllProduct({{current+1}},4)" value="下页"/> <input type="button" v-on:click="findAllProduct('+{{pages}}+',4)" value="末页"/>
您的代码看起来像是一个基于Vue.js的分页功能。根据代码的逻辑推测,您可能希望实现一个上一页、下一页和末页的按钮,并通过调用`findAllProduct`方法来获取相应页码的产品数据。
在上述代码中,使用了Vue.js的指令`v-on:click`来监听按钮的点击事件,并调用了`findAllProduct`方法。您还使用了双花括号`{{}}`来在模板中插入变量。
需要注意的是,双花括号`{{}}`在Vue.js中是用于模板插值的语法,而不应该在事件绑定或表达式中使用。正确的写法应该是将变量直接传递给方法,而不需要使用双花括号。
以下是修正后的代码示例:
```html
<input type="button" v-on:click="findAllProduct(current - 1, 4)" value="上页"/>
<input type="button" v-on:click="findAllProduct(current + 1, 4)" value="下页"/>
<input type="button" v-on:click="findAllProduct(pages, 4)" value="末页"/>
```
请注意,修正后的代码假设您已经在Vue实例中定义了`current`和`pages`这两个变量,并正确实现了`findAllProduct`方法来获取相应页码的产品数据。
<h1>通讯录</h1> <button class="add-btn">添加联系人</button> <input type="text" id="search-input" placeholder="搜索姓名或电话"> <table> <thead> <tr> <th>姓名</th> <th>电话</th> <th>邮箱</th> <th>操作</th> </tr> </thead> <tbody id="contact-list"> <!-- 数据渲染 --> </tbody> </table> <!-- 分页按钮 --> <div id="page-buttons"></div> <!-- 弹出层,用于添加或编辑联系人 --> <div id="modal" class="modal" style="display: none;"> <div class="modal-content"> <span class="close">×</span> <form> <div class="form-group"> <label for="name">姓名</label> <input type="text" id="name" name="name" required> </div> <div class="form-group"> <label for="phone">电话</label> <input type="text" id="phone" name="phone" required> </div> <div class="form-group"> <label for="email">邮箱</label> <input type="text" id="email" name="email" required> </div> <button id="save-btn">保存</button> <button id="cancel-btn">取消</button> </form> </div> </div>帮我加入一个搜索框,点击搜索按钮可以查找到查找的内容;让数据具有添加删除和编辑的功能,编辑的时候增加弹窗进行编辑;添加翻页的功能,每一页最多存放五条数据
好的,以下是加入搜索框、添加删除和编辑功能、以及翻页功能的完整代码:
```
<h1>通讯录</h1>
<button class="add-btn">添加联系人</button>
<input type="text" id="search-input" placeholder="搜索姓名或电话">
<table>
<thead>
<tr>
<th>姓名</th>
<th>电话</th>
<th>邮箱</th>
<th>操作</th>
</tr>
</thead>
<tbody id="contact-list">
<!-- 数据渲染 -->
</tbody>
</table>
<!-- 分页按钮 -->
<div id="page-buttons"></div>
<!-- 弹出层,用于添加或编辑联系人 -->
<div id="modal" class="modal" style="display: none;">
<div class="modal-content">
<span class="close">×</span>
<form>
<div class="form-group">
<label for="name">姓名</label>
<input type="text" id="name" name="name" required>
</div>
<div class="form-group">
<label for="phone">电话</label>
<input type="text" id="phone" name="phone" required>
</div>
<div class="form-group">
<label for="email">邮箱</label>
<input type="text" id="email" name="email" required>
</div>
<button id="save-btn">保存</button>
<button id="cancel-btn">取消</button>
</form>
</div>
</div>
<script>
// 初始化通讯录数组
let contactData = [
{ name: '张三', phone: '123456789', email: 'zhangsan@example.com' },
{ name: '李四', phone: '987654321', email: 'lisi@example.com' },
{ name: '王五', phone: '456123789', email: 'wangwu@example.com' },
{ name: '赵六', phone: '789654123', email: 'zhaoliu@example.com' },
{ name: '钱七', phone: '321456987', email: 'qianqi@example.com' },
{ name: '孙八', phone: '654321987', email: 'sunba@example.com' },
{ name: '周九', phone: '159753456', email: 'zhoujiu@example.com' },
{ name: '吴十', phone: '753159456', email: 'wushi@example.com' },
];
// 每页显示的数据条数
const pageSize = 5;
// 当前页码
let currentPage = 1;
// 根据当前页码渲染数据
function renderData() {
const startIndex = (currentPage - 1) * pageSize;
const endIndex = currentPage * pageSize;
const dataToRender = contactData.slice(startIndex, endIndex);
let html = '';
dataToRender.forEach((contact, index) => {
html += `
<tr>
<td>${contact.name}</td>
<td>${contact.phone}</td>
<td>${contact.email}</td>
<td>
<button class="edit-btn" data-index="${startIndex + index}">编辑</button>
<button class="delete-btn" data-index="${startIndex + index}">删除</button>
</td>
</tr>
`;
});
document.querySelector('#contact-list').innerHTML = html;
}
// 根据当前页码渲染分页按钮
function renderPageButtons() {
const totalPage = Math.ceil(contactData.length / pageSize);
let html = '';
for (let i = 1; i <= totalPage; i++) {
html += `<button class="page-btn ${i === currentPage ? 'active' : ''}" data-page="${i}">${i}</button>`;
}
document.querySelector('#page-buttons').innerHTML = html;
}
// 根据搜索框输入的值筛选数据
function searchContacts() {
const searchInput = document.querySelector('#search-input').value.trim().toLowerCase();
if (!searchInput) {
currentPage = 1;
renderData();
renderPageButtons();
return;
}
const filteredData = contactData.filter(contact => {
return contact.name.toLowerCase().includes(searchInput) || contact.phone.toLowerCase().includes(searchInput);
});
if (filteredData.length === 0) {
document.querySelector('#contact-list').innerHTML = '<tr><td colspan="4">没有找到匹配的联系人</td></tr>';
document.querySelector('#page-buttons').innerHTML = '';
return;
}
contactData = filteredData;
currentPage = 1;
renderData();
renderPageButtons();
}
// 添加联系人弹窗
const modal = document.querySelector('#modal');
const addBtn = document.querySelector('.add-btn');
const closeBtn = document.querySelector('.close');
const saveBtn = document.querySelector('#save-btn');
const cancelBtn = document.querySelector('#cancel-btn');
const nameInput = document.querySelector('#name');
const phoneInput = document.querySelector('#phone');
const emailInput = document.querySelector('#email');
function openModal() {
nameInput.value = '';
phoneInput.value = '';
emailInput.value = '';
modal.style.display = 'block';
}
function closeModal() {
modal.style.display = 'none';
}
function saveContact() {
const name = nameInput.value.trim();
const phone = phoneInput.value.trim();
const email = emailInput.value.trim();
if (!name || !phone || !email) {
alert('请填写完整信息');
return;
}
contactData.push({ name, phone, email });
closeModal();
searchContacts();
}
addBtn.addEventListener('click', openModal);
closeBtn.addEventListener('click', closeModal);
cancelBtn.addEventListener('click', closeModal);
saveBtn.addEventListener('click', saveContact);
// 编辑联系人弹窗
let editingIndex = null;
function openEditModal(index) {
const contact = contactData[index];
nameInput.value = contact.name;
phoneInput.value = contact.phone;
emailInput.value = contact.email;
modal.style.display = 'block';
editingIndex = index;
}
function saveEditedContact() {
const name = nameInput.value.trim();
const phone = phoneInput.value.trim();
const email = emailInput.value.trim();
if (!name || !phone || !email) {
alert('请填写完整信息');
return;
}
contactData[editingIndex] = { name, phone, email };
editingIndex = null;
closeModal();
searchContacts();
}
document.querySelector('#contact-list').addEventListener('click', event => {
if (event.target.matches('.edit-btn')) {
const index = parseInt(event.target.dataset.index);
openEditModal(index);
} else if (event.target.matches('.delete-btn')) {
const index = parseInt(event.target.dataset.index);
contactData.splice(index, 1);
searchContacts();
}
});
// 翻页
document.querySelector('#page-buttons').addEventListener('click', event => {
if (event.target.matches('.page-btn')) {
currentPage = parseInt(event.target.dataset.page);
renderData();
renderPageButtons();
}
});
// 初始化页面
searchContacts();
</script>
```
相关推荐
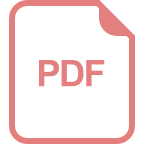
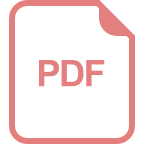
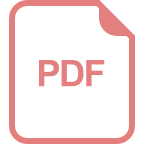












