wordData = wordData.append(data3.ignore_index=True)
时间: 2024-03-02 20:49:57 浏览: 95
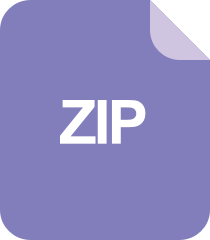
单链表SingleLinkedList.zip_data structure
这段代码有语法错误。正确的写法应该是:
```
wordData = wordData.append(data3, ignore_index=True)
```
这行代码的作用是将`data3`这个数据框添加到`wordData`这个数据框的末尾,并忽略`data3`中原有的索引,重新生成新的索引。`ignore_index=True`的作用就是实现这一功能。
阅读全文
相关推荐
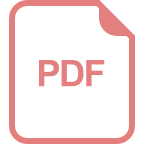
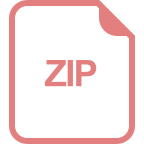



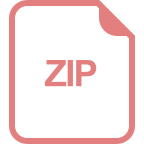
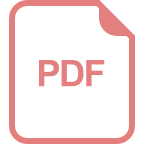
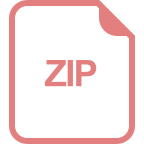
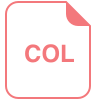
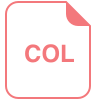
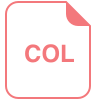







