python简单画图代码
时间: 2023-10-14 07:23:15 浏览: 166
这里提供两种常用的Python画图库:matplotlib和seaborn。下面分别给出简单画图的示例代码。
使用matplotlib画图:
```python
import matplotlib.pyplot as plt
# 生成数据
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# 绘制折线图
plt.plot(x, y)
# 设置图形标题和坐标轴标签
plt.title("Title")
plt.xlabel("X label")
plt.ylabel("Y label")
# 显示图形
plt.show()
```
使用seaborn画图:
```python
import seaborn as sns
# 生成数据
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# 绘制散点图
sns.scatterplot(x, y)
# 设置图形标题和坐标轴标签
plt.title("Title")
plt.xlabel("X label")
plt.ylabel("Y label")
# 显示图形
plt.show()
```
以上代码仅为示例,实际应用中需要根据具体的需求和数据进行调整。
相关问题
python turtle 画图代码
以下是一个简单的Python Turtle画图代码的例子:
```python
import turtle
# 创建一个画布和海龟对象
canvas = turtle.Screen()
t = turtle.Turtle()
# 画一个正方形
for i in range(4):
t.forward(100)
t.right(90)
# 画一个圆形
t.circle(50)
# 改变海龟的颜色和形状
t.color('red', 'yellow')
t.begin_fill()
for i in range(3):
t.forward(100)
t.left(120)
t.end_fill()
# 关闭画布
canvas.exitonclick()
```
这个例子中,我们首先导入了turtle模块,然后创建了一个画布和一个海龟对象。接着,我们使用for循环和turtle的forward()和right()方法画了一个正方形。然后,我们使用circle()方法画了一个圆形。最后,我们改变了海龟的颜色和形状,并使用begin_fill()和end_fill()方法填充了一个三角形。最后,我们关闭了画布。
python搞笑画图代码
以下是一个简单的 Python 画图程序,可以画出一个笑脸:
```python
import turtle
# 画圆函数
def draw_circle(x, y, radius, color):
turtle.up()
turtle.goto(x, y - radius)
turtle.down()
turtle.fillcolor(color)
turtle.begin_fill()
turtle.circle(radius)
turtle.end_fill()
# 画眼睛函数
def draw_eye(x, y, radius):
turtle.up()
turtle.goto(x, y - radius)
turtle.down()
turtle.fillcolor("black")
turtle.begin_fill()
turtle.circle(radius)
turtle.end_fill()
# 画笑嘴函数
def draw_smile(x, y, radius):
turtle.up()
turtle.goto(x - radius, y - radius)
turtle.down()
turtle.setheading(-60)
turtle.circle(radius, 120)
# 画笑脸
draw_circle(0, 0, 100, "yellow")
draw_eye(-40, 50, 10)
draw_eye(40, 50, 10)
draw_smile(0, 0, 70)
turtle.done()
```
运行程序后,将会出现一个笑脸的图形。
阅读全文
相关推荐
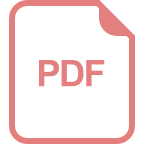



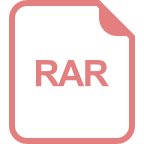
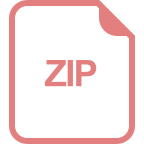







