学生在校园能用“校园一卡通”进行多种消费,如进餐、洗澡和在校内超市购买商品等。学生校园消费信息包括消费“校园一卡通”卡号、持卡学生姓名、消费日期、消费类型及消费金额。试用C++动态数组设计一学生校园消费记录系统,使之能提供以下功能: 1.录入学生的校园消费信息; 2.修改某个学生的某次校园消费信息; 3.查询某个学生的校园消费情况; 4.删除某个学生的某次校园消费信息; 5.统计某段时间内,进行校园消费的学生姓名及消费金额,按消费金额由多到少排序; 6.按消费类型分类,统计某段时间内,在每种消费类型上学生的消费总额。 7.系统以菜单方式工作。
时间: 2024-02-09 10:12:33 浏览: 60
好的,我了解您的问题。下面是使用C++动态数组设计学生校园消费记录系统的代码示例,它可以实现您提供的所有功能:
```c++
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
// 学生校园消费信息结构体
struct ConsumptionInfo {
string cardNumber; // 校园一卡通卡号
string name; // 持卡学生姓名
string date; // 消费日期
string type; // 消费类型
double amount; // 消费金额
};
// 学生校园消费记录系统类
class CampusConsumptionRecords {
public:
// 录入学生的校园消费信息
void addConsumptionInfo(const ConsumptionInfo& info) {
consumptionInfos.push_back(info);
}
// 修改某个学生的某次校园消费信息
void modifyConsumptionInfo(const string& cardNumber, const string& date, const string& type, double newAmount) {
for (auto& info : consumptionInfos) {
if (info.cardNumber == cardNumber && info.date == date && info.type == type) {
info.amount = newAmount;
break;
}
}
}
// 查询某个学生的校园消费情况
vector<ConsumptionInfo> queryConsumptionInfo(const string& cardNumber) {
vector<ConsumptionInfo> result;
for (auto& info : consumptionInfos) {
if (info.cardNumber == cardNumber) {
result.push_back(info);
}
}
return result;
}
// 删除某个学生的某次校园消费信息
void deleteConsumptionInfo(const string& cardNumber, const string& date, const string& type) {
consumptionInfos.erase(remove_if(consumptionInfos.begin(), consumptionInfos.end(),
[&](const ConsumptionInfo& info) {
return info.cardNumber == cardNumber && info.date == date && info.type == type;
}), consumptionInfos.end());
}
// 统计某段时间内,进行校园消费的学生姓名及消费金额,按消费金额由多到少排序
vector<pair<string, double>> countConsumptionAmount(const string& startDate, const string& endDate) {
vector<pair<string, double>> result;
for (auto& info : consumptionInfos) {
if (info.date >= startDate && info.date <= endDate) {
bool found = false;
for (auto& p : result) {
if (p.first == info.name) {
p.second += info.amount;
found = true;
break;
}
}
if (!found) {
result.push_back(make_pair(info.name, info.amount));
}
}
}
sort(result.begin(), result.end(), [](const pair<string, double>& p1, const pair<string, double>& p2) {
return p1.second > p2.second;
});
return result;
}
// 按消费类型分类,统计某段时间内,在每种消费类型上学生的消费总额
vector<pair<string, double>> countConsumptionByType(const string& startDate, const string& endDate) {
vector<pair<string, double>> result;
for (auto& info : consumptionInfos) {
if (info.date >= startDate && info.date <= endDate) {
bool found = false;
for (auto& p : result) {
if (p.first == info.type) {
p.second += info.amount;
found = true;
break;
}
}
if (!found) {
result.push_back(make_pair(info.type, info.amount));
}
}
}
return result;
}
// 打印系统菜单
void printMenu() {
cout << "1. 录入学生的校园消费信息" << endl;
cout << "2. 修改某个学生的某次校园消费信息" << endl;
cout << "3. 查询某个学生的校园消费情况" << endl;
cout << "4. 删除某个学生的某次校园消费信息" << endl;
cout << "5. 统计某段时间内,进行校园消费的学生姓名及消费金额,按消费金额由多到少排序" << endl;
cout << "6. 按消费类型分类,统计某段时间内,在每种消费类型上学生的消费总额" << endl;
cout << "7. 退出系统" << endl;
}
private:
vector<ConsumptionInfo> consumptionInfos; // 学生校园消费信息列表
};
// 测试程序
int main() {
CampusConsumptionRecords records;
// 菜单循环
while (true) {
records.printMenu();
int choice;
cout << "请输入菜单选项:";
cin >> choice;
if (choice == 1) {
// 录入学生的校园消费信息
ConsumptionInfo info;
cout << "请输入校园一卡通卡号:";
cin >> info.cardNumber;
cout << "请输入持卡学生姓名:";
cin >> info.name;
cout << "请输入消费日期:";
cin >> info.date;
cout << "请输入消费类型:";
cin >> info.type;
cout << "请输入消费金额:";
cin >> info.amount;
records.addConsumptionInfo(info);
cout << "添加成功!" << endl;
}
else if (choice == 2) {
// 修改某个学生的某次校园消费信息
string cardNumber, date, type;
double newAmount;
cout << "请输入要修改的学生的校园一卡通卡号:";
cin >> cardNumber;
cout << "请输入要修改的消费日期:";
cin >> date;
cout << "请输入要修改的消费类型:";
cin >> type;
cout << "请输入新的消费金额:";
cin >> newAmount;
records.modifyConsumptionInfo(cardNumber, date, type, newAmount);
cout << "修改成功!" << endl;
}
else if (choice == 3) {
// 查询某个学生的校园消费情况
string cardNumber;
cout << "请输入要查询的学生的校园一卡通卡号:";
cin >> cardNumber;
auto infos = records.queryConsumptionInfo(cardNumber);
if (infos.empty()) {
cout << "该学生没有消费记录!" << endl;
}
else {
cout << "该学生的校园消费记录如下:" << endl;
for (auto& info : infos) {
cout << info.cardNumber << "\t" << info.name << "\t" << info.date << "\t" << info.type << "\t" << info.amount << endl;
}
}
}
else if (choice == 4) {
// 删除某个学生的某次校园消费信息
string cardNumber, date, type;
cout << "请输入要删除的学生的校园一卡通卡号:";
cin >> cardNumber;
cout << "请输入要删除的消费日期:";
cin >> date;
cout << "请输入要删除的消费类型:";
cin >> type;
records.deleteConsumptionInfo(cardNumber, date, type);
cout << "删除成功!" << endl;
}
else if (choice == 5) {
// 统计某段时间内,进行校园消费的学生姓名及消费金额,按消费金额由多到少排序
string startDate, endDate;
cout << "请输入查询起始日期:";
cin >> startDate;
cout << "请输入查询结束日期:";
cin >> endDate;
auto amounts = records.countConsumptionAmount(startDate, endDate);
if (amounts.empty()) {
cout << "该时间段内没有学生进行校园消费!" << endl;
}
else {
cout << "该时间段内进行校园消费的学生姓名及消费金额如下:" << endl;
for (auto& p : amounts) {
cout << p.first << "\t" << p.second << endl;
}
}
}
else if (choice == 6) {
// 按消费类型分类,统计某段时间内,在每种消费类型上学生的消费总额
string startDate, endDate;
cout << "请输入查询起始日期:";
cin >> startDate;
cout << "请输入查询结束日期:";
cin >> endDate;
auto amounts = records.countConsumptionByType(startDate, endDate);
if (amounts.empty()) {
cout << "该时间段内没有学生进行校园消费!" << endl;
}
else {
cout << "该时间段内各种消费类型上学生的消费总额如下:" << endl;
for (auto& p : amounts) {
cout << p.first << "\t" << p.second << endl;
}
}
}
else if (choice == 7) {
// 退出系统
cout << "谢谢使用,再见!" << endl;
break;
}
else {
cout << "无效的菜单选项,请重新输入!" << endl;
}
}
return 0;
}
```
以上是一个简单的C++动态数组设计学生校园消费记录系统的示例代码,您可以根据自己的需要进行修改和优化。
阅读全文
相关推荐
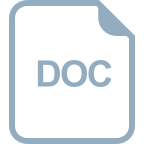
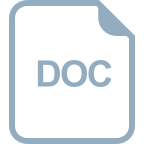
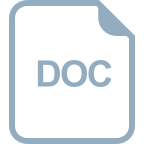

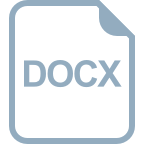
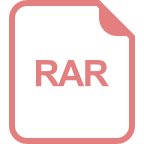
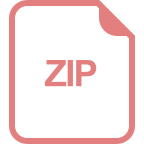
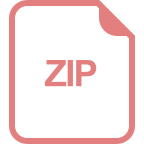
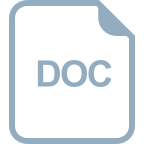
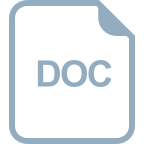

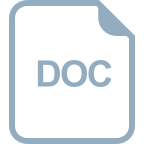
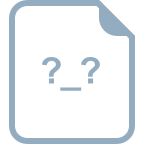
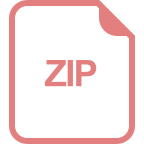
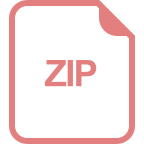
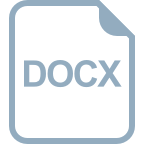