java学生持有校园一卡通在多个校区不同地点(食堂、教育超市、浴室、自动售卖机等)进行消费。学生要关注自己的消费情况,比如某段时间的消费总额、消费的地点分布等信息,该如何进行类及其关系的设计?对上述场景进行类及其关系的设计,并给出设计的类的具体实现。
时间: 2024-02-15 15:03:14 浏览: 35
根据上述场景,可以设计以下类及其关系:
1. 学生类(Student)
属性:学号(id)、姓名(name)、校园一卡通(card)
方法:获取消费记录(getRecords)
2. 消费记录类(Record)
属性:消费金额(amount)、消费地点(location)、消费时间(time)
3. 校园一卡通类(CampusCard)
属性:余额(balance)、消费记录(records)
方法:添加消费记录(addRecord)、获取消费记录(getRecords)
4. 消费地点类(Location)
属性:地点名称(name)
5. 地点记录类(LocationRecord)
属性:地点名称(location)、消费次数(count)、消费金额(amount)
6. 时间段类(TimeRange)
属性:起始时间(startTime)、结束时间(endTime)
方法:获取消费记录(getRecords)
7. 校区类(Campus)
属性:校区名称(name)、地点列表(locations)
方法:添加地点(addLocation)、获取地点记录(getLocationRecords)
类的具体实现:
```
public class Student {
private String id;
private String name;
private CampusCard card;
public Student(String id, String name, CampusCard card) {
this.id = id;
this.name = name;
this.card = card;
}
public List<Record> getRecords() {
return card.getRecords();
}
}
public class Record {
private double amount;
private Location location;
private Date time;
public Record(double amount, Location location, Date time) {
this.amount = amount;
this.location = location;
this.time = time;
}
}
public class CampusCard {
private double balance;
private List<Record> records;
public CampusCard(double balance) {
this.balance = balance;
this.records = new ArrayList<>();
}
public void addRecord(Record record) {
this.records.add(record);
this.balance -= record.getAmount();
}
public List<Record> getRecords() {
return this.records;
}
}
public class Location {
private String name;
public Location(String name) {
this.name = name;
}
}
public class LocationRecord {
private Location location;
private int count;
private double amount;
public LocationRecord(Location location, int count, double amount) {
this.location = location;
this.count = count;
this.amount = amount;
}
}
public class TimeRange {
private Date startTime;
private Date endTime;
public TimeRange(Date startTime, Date endTime) {
this.startTime = startTime;
this.endTime = endTime;
}
public List<Record> getRecords(CampusCard card) {
List<Record> records = card.getRecords();
List<Record> filteredRecords = new ArrayList<>();
for (Record record : records) {
if (record.getTime().after(this.startTime) && record.getTime().before(this.endTime)) {
filteredRecords.add(record);
}
}
return filteredRecords;
}
}
public class Campus {
private String name;
private List<Location> locations;
public Campus(String name) {
this.name = name;
this.locations = new ArrayList<>();
}
public void addLocation(Location location) {
this.locations.add(location);
}
public List<LocationRecord> getLocationRecords(TimeRange timeRange) {
List<LocationRecord> locationRecords = new ArrayList<>();
for (Location location : locations) {
int count = 0;
double amount = 0;
for (Record record : location.getRecords()) {
if (timeRange.getRecords(record.getCard()).contains(record)) {
count++;
amount += record.getAmount();
}
}
LocationRecord locationRecord = new LocationRecord(location, count, amount);
locationRecords.add(locationRecord);
}
return locationRecords;
}
}
```
相关推荐
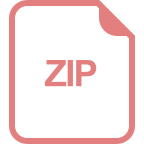
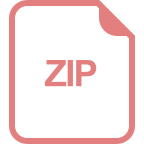
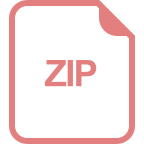














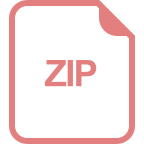