vue实现拖拽产生连线的代码
时间: 2023-06-12 13:07:30 浏览: 117
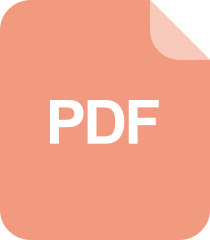
vue使用drag与drop实现拖拽的示例代码
以下是一个用 Vue 实现拖拽产生连线的示例代码:
```html
<template>
<div class="container">
<div class="box" v-for="(item, index) in boxes" :key="index" :style="{ top: item.top + 'px', left: item.left + 'px' }" @mousedown="startDrag($event, item)">
<span>{{ index }}</span>
</div>
<svg class="line-container">
<line v-for="(line, index) in lines" :key="index" :x1="line.x1" :y1="line.y1" :x2="line.x2" :y2="line.y2" />
</svg>
</div>
</template>
<script>
export default {
data() {
return {
boxes: [
{ top: 50, left: 50 },
{ top: 50, left: 200 },
{ top: 200, left: 50 },
{ top: 200, left: 200 }
],
lines: []
}
},
methods: {
startDrag(event, item) {
const startX = event.pageX
const startY = event.pageY
const startTop = item.top
const startLeft = item.left
const moveHandler = (event) => {
const deltaX = event.pageX - startX
const deltaY = event.pageY - startY
item.top = startTop + deltaY
item.left = startLeft + deltaX
}
const upHandler = () => {
document.removeEventListener('mousemove', moveHandler)
document.removeEventListener('mouseup', upHandler)
this.updateLines()
}
document.addEventListener('mousemove', moveHandler)
document.addEventListener('mouseup', upHandler)
},
updateLines() {
const boxes = this.$el.querySelectorAll('.box')
const lines = []
for (let i = 0; i < boxes.length; i++) {
const box1 = boxes[i]
const box1Rect = box1.getBoundingClientRect()
for (let j = i + 1; j < boxes.length; j++) {
const box2 = boxes[j]
const box2Rect = box2.getBoundingClientRect()
const line = {
x1: box1Rect.left + box1Rect.width / 2,
y1: box1Rect.top + box1Rect.height / 2,
x2: box2Rect.left + box2Rect.width / 2,
y2: box2Rect.top + box2Rect.height / 2
}
lines.push(line)
}
}
this.lines = lines
}
}
}
</script>
<style>
.container {
position: relative;
width: 400px;
height: 400px;
background-color: #f1f1f1;
}
.box {
position: absolute;
width: 50px;
height: 50px;
background-color: #fff;
border: 1px solid #ddd;
display: flex;
justify-content: center;
align-items: center;
cursor: move;
}
.box span {
font-size: 20px;
}
.line-container {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
pointer-events: none;
}
</style>
```
这里的核心思路是,当鼠标按下时记录当前元素的位置,然后在鼠标移动时计算偏移量,从而更新元素的位置。每次更新位置时,都重新计算连线的位置。具体实现方法是,使用 `getBoundingClientRect()` 方法获取元素的位置和尺寸,然后计算两个元素的中心点坐标,从而确定连线的起点和终点坐标。最后用 SVG 的 `line` 元素来绘制连线。
阅读全文
相关推荐
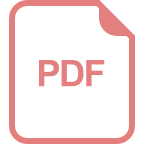
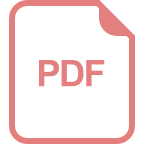

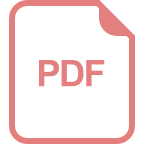
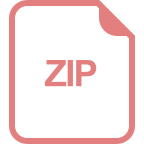







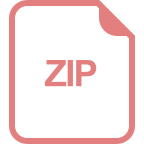
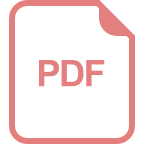
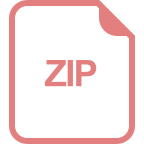
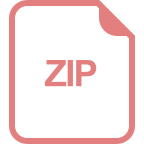

