HttpMethod get =new Getmethod(param); get.getparams().setcontentcharset("UTF-8"); int statusCode; statusCode =httpclient.executeMethod(get); String bodyString =get.getResponseBodyAsString(); 上面是一段Java代码请按步骤详细解释并说明是否合理并给出理由
时间: 2023-06-15 12:05:25 浏览: 170
这段Java代码的功能是使用HttpClient库发送一个HTTP GET请求,并获取响应的HTTP状态码和响应体。
具体解释如下:
1. 第一行代码创建了一个HttpGet对象,该对象的构造方法需要传入一个URL参数,用于指定要发送请求的目标地址。
2. 第二行代码调用了HttpGet对象的getParams()方法,获取请求参数对象,然后使用setContentCharset()方法设置请求体的字符集为UTF-8。这一步是可选的,如果请求体中不包含中文或其他非ASCII字符,可以省略这一步。
3. 第三行代码创建了一个HttpClient对象,用于发送HTTP请求。
4. 第四行代码执行了HTTP GET请求,并将响应的HTTP状态码保存在statusCode变量中。
5. 第五行代码调用了HttpGet对象的getResponseBodyAsString()方法,获取响应体的字符串表示。
这段Java代码看起来是合理的,因为它使用了HttpClient库提供的标准API,而且按照常规的HTTP请求流程发送了一个GET请求并获取响应。唯一需要注意的是,如果请求的URL包含了特殊字符(比如空格、中文、特殊符号等),需要进行URL编码,否则可能会导致请求失败。
相关问题
Java中httpmethod get=new getmethod(param)
在Java中,使用Apache HttpClient库可以发送http请求。其中,GET请求可以通过创建HttpGet实例来实现,具体代码如下:
```
HttpGet httpGet = new HttpGet(url + "?" + param); // 创建HttpGet实例
HttpResponse httpResponse = httpClient.execute(httpGet); // 发送http请求并接收响应
```
其中,url是请求的地址,param是请求所携带的参数。需要注意的是,param需要按照key1=value1&key2=value2的格式进行拼接。例如:
```
String url = "http://example.com/api";
String param = "id=123&name=Tom";
HttpGet httpGet = new HttpGet(url + "?" + param); // 创建HttpGet实例
HttpResponse httpResponse = httpClient.execute(httpGet); // 发送http请求并接收响应
```
这段代码会向http://example.com/api发送一个GET请求,请求参数为id=123&name=Tom。具体的http请求的响应可以通过HttpResponse对象获取。
解释 public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception { //支持跨域请求 response.setHeader("Access-Control-Allow-Methods", "POST, GET, OPTIONS, DELETE"); response.setHeader("Access-Control-Max-Age", "3600"); response.setHeader("Access-Control-Allow-Credentials", "true"); response.setHeader("Access-Control-Allow-Headers", "x-requested-with,request-source,Token, Origin,imgType, Content-Type, cache-control,postman-token,Cookie, Accept,authorization"); response.setHeader("Access-Control-Allow-Origin", request.getHeader("Origin")); // 跨域时会首先发送一个OPTIONS请求,这里我们给OPTIONS请求直接返回正常状态 if (request.getMethod().equals(RequestMethod.OPTIONS.name())) { response.setStatus(HttpStatus.OK.value()); return false; } IgnoreAuth annotation; if (handler instanceof HandlerMethod) { annotation = ((HandlerMethod) handler).getMethodAnnotation(IgnoreAuth.class); } else { return true; } //从header中获取token String token = request.getHeader(LOGIN_TOKEN_KEY); /** * 不需要验证权限的方法直接放过 */ if(annotation!=null) { return true; } TokenEntity tokenEntity = null; if(StringUtils.isNotBlank(token)) { tokenEntity = tokenService.getTokenEntity(token); } if(tokenEntity != null) { request.getSession().setAttribute("userId", tokenEntity.getUserid()); request.getSession().setAttribute("role", tokenEntity.getRole()); request.getSession().setAttribute("tableName", tokenEntity.getTablename()); request.getSession().setAttribute("username", tokenEntity.getUsername()); return true; } PrintWriter writer = null; response.setCharacterEncoding("UTF-8"); response.setContentType("application/json; charset=utf-8"); try { writer = response.getWriter(); writer.print(JSONObject.toJSONString(R.error(401, "请先登录"))); } finally { if(writer != null){ writer.close(); } } // throw new EIException("请先登录", 401); return false; }
这段代码是一个Java的拦截器,用于对请求进行预处理。在这个方法中,首先设置了支持跨域请求的相关参数,然后判断是否为OPTIONS请求,如果是则直接返回正常状态。接下来判断是否有IgnoreAuth注解,如果有则直接放过,否则从请求头中获取Token,并通过TokenService验证Token的有效性。如果Token有效,则将相关信息存入Session中,否则返回401错误提示。最后,返回true或者false表示是否允许请求继续处理。
阅读全文
相关推荐
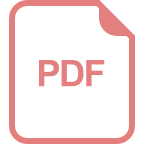
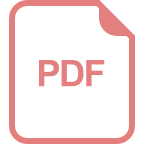
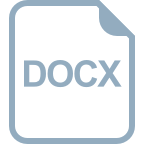










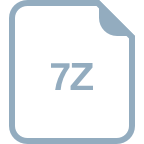
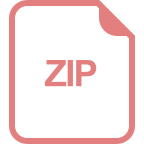
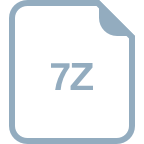