matplotlib.pyplot.bar参数百分比
时间: 2023-10-25 13:14:27 浏览: 153
如果您想在 matplotlib.pyplot.bar 中使用百分比,可以将 y 值除以总和,并使用 yticks 和 yticklabels 来设置标签。以下是一个例子:
```python
import matplotlib.pyplot as plt
# 一些示例数据
values = [25, 35, 20, 10, 10]
# 计算总和
total = sum(values)
# 将值转换为百分比
percentages = [100 * value / total for value in values]
# 绘制条形图
plt.bar(range(len(values)), percentages)
# 设置刻度标签
plt.yticks(range(0, 110, 10), ['{}%'.format(x) for x in range(0, 110, 10)])
plt.show()
```
在上面的代码中,我们首先计算了值的总和,然后将每个值转换为百分比,并使用 `plt.bar()` 绘制了条形图。最后,我们使用 `plt.yticks()` 设置了刻度标签,其中第一个参数是刻度位置,第二个参数是对应的标签列表。我们使用列表推导式来生成标签列表,其中每个标签都是一个字符串,包含百分比值。
相关问题
对文件profit.xls中的盈利数据做出 帕累托图(帕累托图的定义可百度),用matplotlib.pyplot作图,写出代码。
帕累托图是一种图形工具,用于显示项目按照重要性排序的比例分布。在这种情况下,我们将根据利润数据制作一个帕累托图,以展示数据集中盈利最高的部分占比情况。这里是一个简单的Python代码示例,使用matplotlib.pyplot库:
```python
import pandas as pd
import matplotlib.pyplot as plt
# 读取profit.xls文件中的数据
data = pd.read_excel('profit.xls')
# 假设利润列名为'Profit'
profits = data['Profit']
# 计算累积频率(百分比)
cumulative_profits = profits.value_counts().sort_values(ascending=False).cumsum() / profits.sum()
# 创建帕累托图
plt.figure(figsize=(10, 6))
plt.plot(cumulative_profits.index, cumulative_profits, marker='o')
plt.xlabel('利润类别')
plt.ylabel('累计比例')
plt.title('利润数据的帕累托图')
plt.grid(True)
plt.xticks(rotation=45) # 根据需要调整x轴标签角度
plt.fill_between(cumulative_profits.index, 0, cumulative_profits, alpha=0.5) # 阴影部分代表累积比例
# 绘制直方图,与帕累托图一起展示
plt.bar(profits.index, profits, color='skyblue', align='center', alpha=0.5, edgecolor='black')
plt.legend(['累积比例', '利润分布'], loc='upper right')
plt.show()
import matplotlib as mpl import matplotlib.pyplot as plt plt.subplots_adjust(left=None, bottom=None, right=None, top=None, wspace=None, hspace=0.5) t=np.arange(0.0,2.0,0.1) s=np.sin(t*np.pi) plt.subplot(2,2,1) #要生成两行两列,这是第一个图 import numpy as np import matplotlib.pyplot as plt x = np.arange(1,13) y1 = np.array([53673, 57571, 58905, 55239, 49661, 49510, 49163, 57311, 59187, 60074, 57109, 52885]) plt.plot(x, y1) plt.title('近13天登录人数') plt.show() plt.subplot(2,2,2) #两行两列,这是第二个图 import numpy as np import matplotlib.pyplot as plt plt.subplots_adjust(top=0.85) x = np.arange(12) y = np.array([70887, 64957, 62508, 66471, 54972, 46245, 64083, 67090, 64991, 88504, 79404, 68253,]) bar_width = 0.3 plt.bar(x, y, tick_label=['1月', '2月', '3月', '4月', '5月', '6月', '7月', "8月", "9月", "10月", '11月', '12月'],width=bar_width) plt.title('近12个月人数') plt.show() plt.subplot(2,2,3)#两行两列,这是第三个图 import matplotlib.pyplot as plt plt.subplots_adjust(top=0.85) plt.style.use('fivethirtyeight') languages = ['steam', 'ubisoft'] popularity = [78,22] plt.pie(popularity) plt.tight_layout() plt.pie(popularity, labels=languages, autopct='%1.1f%%') plt.title('游戏平台登录占比') plt.subplot(2,2,4)#两行两列,这是第四个图 import numpy as np from matplotlib import pyplot as plt ages_x = [1, 2, 3, 4, 5, ] x_indexes = np.arange(len(ages_x)) width = 0.33 dev_y = [64050, 65168, 75588, 75590, 60097] py_dev_y = [57809, 56101, 70605, 63437, 56756] plt.bar(x_indexes, dev_y, width=width, label="2022") plt.bar(x_indexes + width, py_dev_y, width=width, label="2023") plt.xlabel("月份") plt.ylabel("平均在线人数") plt.title("2022和2023一到五月在线人数对比") plt.legend() plt.xticks(ticks = x_indexes, labels = ages_x) plt.show()
你刚才的代码中,第三个子图中的饼图有一些问题。虽然你使用了pie函数绘制了饼图,但是没有正确地添加标签和百分比。正确的代码应该是这样的:
```python
plt.subplot(2,2,3)
plt.subplots_adjust(top=0.85)
plt.style.use('fivethirtyeight')
languages = ['steam', 'ubisoft']
popularity = [78,22]
plt.pie(popularity, labels=languages, autopct='%1.1f%%')
plt.title('游戏平台登录占比')
plt.show()
```
这样做可以让饼图正确地显示游戏平台的登录占比,并显示出每个部分所占的百分比。
阅读全文
相关推荐
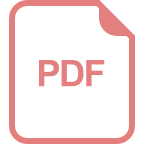



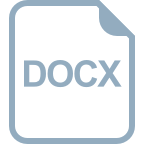
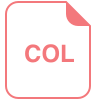
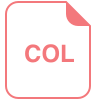
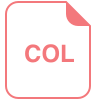
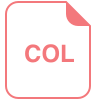
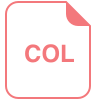
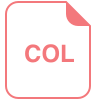



