Java编写程序,运用接口,画出圆形,正方形,三角形
时间: 2024-04-20 21:17:01 浏览: 296
好的,我可以为您提供Java程序的示例代码,用于画出圆形、正方形和三角形。
首先,需要导入Java的绘图包java.awt和javax.swing。然后,可以定义一个接口Shape,其中包括画图方法draw()。接下来,可以定义三个类Circle、Square和Triangle,实现接口Shape并重新定义draw()方法,在方法中使用Java绘图API来画出相应的形状。
示例代码如下:
```java
import java.awt.Graphics;
import javax.swing.JFrame;
import javax.swing.JPanel;
interface Shape {
public void draw(Graphics g);
}
class Circle implements Shape {
int x, y, r;
public Circle(int x, int y, int r) {
this.x = x;
this.y = y;
this.r = r;
}
public void draw(Graphics g) {
g.drawOval(x-r, y-r, 2*r, 2*r);
}
}
class Square implements Shape {
int x, y, side;
public Square(int x, int y, int side) {
this.x = x;
this.y = y;
this.side = side;
}
public void draw(Graphics g) {
g.drawRect(x, y, side, side);
}
}
class Triangle implements Shape {
int[] xPoints = {100, 150, 50};
int[] yPoints = {100, 150, 150};
public void draw(Graphics g) {
g.drawPolygon(xPoints, yPoints, 3);
}
}
class MyPanel extends JPanel {
Shape[] shapes;
public MyPanel() {
shapes = new Shape[3];
shapes[0] = new Circle(100, 100, 50);
shapes[1] = new Square(200, 100, 50);
shapes[2] = new Triangle();
}
public void paint(Graphics g) {
super.paint(g);
for (Shape s : shapes) {
s.draw(g);
}
}
}
public class Main {
public static void main(String[] args) {
JFrame frame = new JFrame("Shapes");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(new MyPanel());
frame.setSize(400, 400);
frame.setVisible(true);
}
}
```
在这个示例程序中,定义了三个类Circle、Square和Triangle,它们都实现了接口Shape,并分别重写了draw()方法,在方法中使用相应的Java绘图API来画出圆形、正方形和三角形,然后在MyPanel类中将它们同时绘制到界面上。最后,在主函数中创建一个JFrame对象来显示界面。
阅读全文
相关推荐
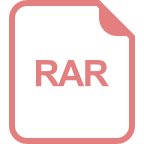















