python实现一个方法,记录鼠标每次点击位置,计算两次点击的时间间隔,调用一个方法读取回放鼠标点击的位置和点击频率
时间: 2023-06-20 07:04:48 浏览: 45
以下是一个简单的Python实现方法,可以记录鼠标点击位置,计算两次点击的时间间隔,并将记录保存到文件中:
```python
import time
import os
import sys
import tkinter as tk
class MouseRecorder:
def __init__(self):
self.positions = [] # 存储鼠标点击位置
self.times = [] # 存储鼠标点击时间
self.last_click_time = None # 上次点击时间
# 创建一个隐藏的窗口用于监听鼠标事件
self.root = tk.Tk()
self.root.withdraw()
self.root.bind('<Button-1>', self.record_click)
def record_click(self, event):
# 记录鼠标点击位置和时间
self.positions.append((event.x, event.y))
self.times.append(time.time())
# 计算时间间隔
if self.last_click_time is not None:
delta_time = self.times[-1] - self.last_click_time
print(f'Time interval: {delta_time:.2f}s')
self.last_click_time = self.times[-1]
def save_recordings(self, filename):
# 将记录保存到文件中
with open(filename, 'w') as f:
for i in range(len(self.positions)):
f.write(f'{self.positions[i][0]},{self.positions[i][1]},{self.times[i]:.6f}\n')
def play_recordings(self, filename, speed=1):
# 读取记录并回放鼠标点击位置
with open(filename, 'r') as f:
lines = f.readlines()
for line in lines:
x, y, t = line.strip().split(',')
x, y, t = int(x), int(y), float(t)
self.root.event_generate('<Button-1>', x=x, y=y)
time.sleep((t - self.times[0]) / speed)
if __name__ == '__main__':
recorder = MouseRecorder()
print('左键单击记录鼠标点击位置,右键单击保存并退出')
while True:
try:
recorder.root.update()
except tk.TclError:
break
if recorder.root.mouse_click:
event_type, event_button = recorder.root.mouse_click
if event_type == 'button-3':
filename = input('请输入保存文件名: ')
recorder.save_recordings(filename)
sys.exit(0)
filename = input('请输入回放文件名: ')
if not os.path.exists(filename):
print('文件不存在')
sys.exit(1)
speed = float(input('请输入回放速度(1为正常速度): '))
recorder.play_recordings(filename, speed)
```
使用方法:
1. 运行上述代码,会弹出一个空白窗口;
2. 在空白窗口上左键单击,程序会记录鼠标点击位置和时间,并在控制台上打印时间间隔;
3. 在空白窗口上右键单击,程序会要求输入保存文件名,并将记录保存到文件中;
4. 输入保存的文件名后程序会退出;
5. 再次运行程序,输入保存的文件名,程序会读取记录并回放鼠标点击位置。可以输入回放速度,1为正常速度。
相关推荐
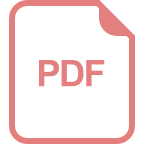
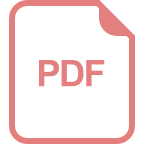
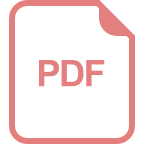














