python实现记录鼠标点击的位置和时间
时间: 2023-06-18 19:06:46 浏览: 308
你可以使用 Python 的 PyAutoGUI 库来记录鼠标点击的位置和时间。PyAutoGUI 是一个允许 Python 控制鼠标和键盘的库。
首先,你需要安装 PyAutoGUI。你可以使用 pip 命令来安装:
```
pip install pyautogui
```
然后,你可以使用以下代码来记录鼠标点击的位置和时间:
```python
import pyautogui
import time
while True:
x, y = pyautogui.position()
print(f'位置:({x}, {y}),时间:{time.time()}')
time.sleep(1)
```
这段代码将不断地输出鼠标位置和当前时间,每秒输出一次。你可以在需要记录鼠标点击位置和时间的时候运行这段代码,并在需要停止记录时按 Ctrl + C 终止程序。
相关问题
python实现记录鼠标点击的位置和下一次点击的时间间隔
你可以使用Python的PyAutoGUI库来实现记录鼠标点击位置和时间间隔的功能。
首先需要安装PyAutoGUI库,可以使用以下命令进行安装:
```
pip install pyautogui
```
然后,可以使用以下代码来记录鼠标点击位置和时间间隔:
```python
import pyautogui
import time
clicks = []
while True:
x, y = pyautogui.position()
clicks.append((x, y, time.time()))
time.sleep(0.1)
if len(clicks) > 1:
interval = clicks[-1][2] - clicks[-2][2]
print(f"Interval: {interval:.2f} seconds")
```
代码中使用了一个无限循环来不断获取鼠标位置,并记录每次点击的位置和时间,存储在一个列表中。当列表中的元素数量大于1时,计算相邻两次点击的时间间隔,并输出到控制台。
需要注意的是,这个程序将会一直运行,直到你手动停止它。你可以按下Ctrl + C来停止程序。
python与tkinter实现鼠标点击与记录鼠标和回放
可以通过Python的Tkinter模块实现鼠标点击、记录鼠标和回放的功能。
以下是一个简单的示例代码,可以记录鼠标点击的位置和时间,并且回放这些点击事件:
```python
import tkinter as tk
import time
class App:
def __init__(self, master):
self.master = master
self.master.bind("<Button-1>", self.click) # 绑定鼠标左键点击事件
self.master.bind("<Button-3>", self.playback) # 绑定鼠标右键点击事件
self.clicks = [] # 用于存储点击事件的列表
def click(self, event):
self.clicks.append((event.x, event.y, time.time())) # 存储点击事件的位置和时间
def playback(self, event):
for click in self.clicks:
x, y, t = click
self.master.after(int((t - self.clicks[0][2]) * 1000), self.move_mouse, x, y) # 模拟鼠标移动
time.sleep(0.1) # 暂停一段时间,以便观察
def move_mouse(self, x, y):
self.master.event_generate("<Motion>", warp=True, x=x, y=y) # 模拟鼠标移动事件
root = tk.Tk()
app = App(root)
root.mainloop()
```
在这个例子中,我们绑定了鼠标左键点击事件和鼠标右键点击事件。当用户点击鼠标左键时,我们将该事件的位置和时间存储在列表中。当用户点击鼠标右键时,我们回放所有存储的点击事件。
回放时,我们使用`self.master.after`函数模拟鼠标移动,`self.move_mouse`函数用于实现鼠标移动。在这个函数中,我们使用`self.master.event_generate`函数生成一个鼠标移动事件。这个事件的位置是我们从列表中读取的,事件的时间是根据前一个事件的时间和当前事件的时间计算得出的。
需要注意的是,在回放时,我们使用了`time.sleep`函数暂停一段时间,以便观察每个点击事件的效果。这个时间可以根据需要进行调整。
阅读全文
相关推荐
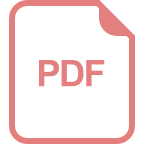
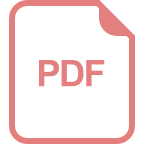
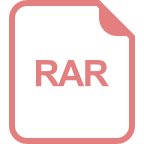













