怎么对多维数组进行顺时针排序
时间: 2023-07-20 13:06:09 浏览: 103
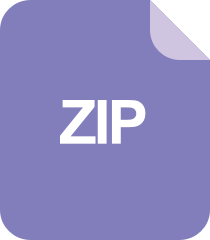
(完整数据)ESG数据大全(论文复刻、彭博、华证、商道融绿、富时罗素等)2022年
对于一个二维数组,可以使用模拟顺时针排序的方法来实现。具体步骤如下:
1. 定义四个变量 `left`、`right`、`top`、`bottom` 分别表示当前未排序部分的左、右、上、下四个边界。
2. 定义一个变量 `direction` 表示当前遍历的方向,初始值为 0,表示从左到右。
3. 使用一个 while 循环不断进行排序,直到左右或者上下两个边界相遇为止。
4. 根据 `direction` 的值,依次遍历当前边界上的元素,并将其加入结果数组中。
5. 遍历完当前边界上的元素后,更新相应的边界和 `direction` 的值,继续进行下一轮遍历。
以下是 Python 代码实现:
```python
def clockwise_sort(matrix):
if not matrix:
return []
m, n = len(matrix), len(matrix[0])
res = []
left, right, top, bottom = 0, n - 1, 0, m - 1
direction = 0
while left <= right and top <= bottom:
if direction == 0:
for i in range(left, right + 1):
res.append(matrix[top][i])
top += 1
elif direction == 1:
for i in range(top, bottom + 1):
res.append(matrix[i][right])
right -= 1
elif direction == 2:
for i in range(right, left - 1, -1):
res.append(matrix[bottom][i])
bottom -= 1
else:
for i in range(bottom, top - 1, -1):
res.append(matrix[i][left])
left += 1
direction = (direction + 1) % 4
return res
```
该算法的时间复杂度为 $O(mn)$,其中 $m$ 和 $n$ 分别为数组的行数和列数。
阅读全文
相关推荐
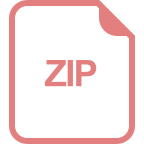
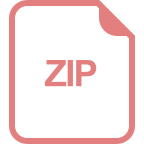
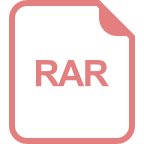
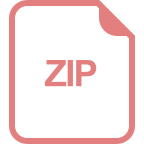
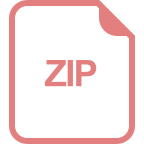
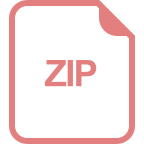
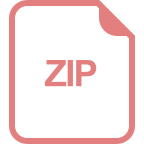
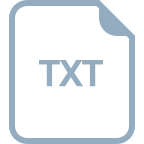
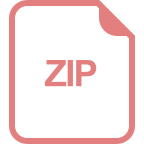
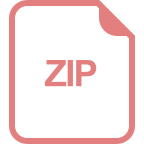
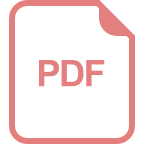
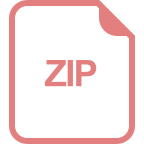
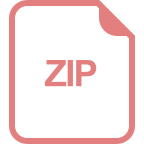
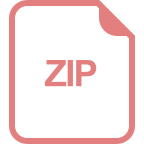
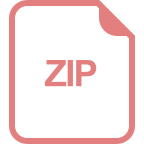