链表插入结点(在查找到的结点之后插入)C语言
时间: 2023-11-06 20:06:58 浏览: 81
链表插入结点的基本思路是:先找到要插入的位置(即要插入结点的前一个结点),然后将插入结点链接到该位置的下一个结点,同时将该位置的指针指向插入结点。下面是在查找到的结点之后插入结点的C语言代码实现:
```c
typedef struct Node {
int data; // 数据域
struct Node *next; // 指针域
} Node;
// 在链表中查找指定值的结点
Node* searchNode(Node *head, int value) {
Node *p = head->next;
while (p != NULL) {
if (p->data == value) {
return p; // 返回查找到的结点
}
p = p->next;
}
return NULL; // 没有找到,返回NULL
}
// 在查找到的结点之后插入结点
void insertAfter(Node *pNode, int value) {
Node *newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = pNode->next;
pNode->next = newNode;
}
int main() {
Node *head = (Node*)malloc(sizeof(Node)); // 创建头结点
head->next = NULL; // 初始化为空链表
// 尾插法创建链表
Node *tail = head;
for (int i = 1; i <= 5; i++) {
Node *newNode = (Node*)malloc(sizeof(Node));
newNode->data = i;
newNode->next = NULL;
tail->next = newNode;
tail = newNode;
}
// 在链表中查找指定值的结点
Node *pNode = searchNode(head, 3);
if (pNode != NULL) {
// 在查找到的结点之后插入结点
insertAfter(pNode, 6);
}
// 遍历链表
Node *p = head->next;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
// 释放链表内存
Node *pNode = head->next;
while (pNode != NULL) {
Node *temp = pNode;
pNode = pNode->next;
free(temp);
}
free(head);
return 0;
}
```
上述代码中,先通过 `searchNode` 函数在链表中查找指定值的结点,然后调用 `insertAfter` 函数在查找到的结点之后插入新结点。最后,通过遍历链表输出每个结点的值,然后释放链表内存。
阅读全文
相关推荐
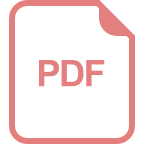


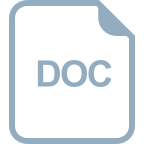
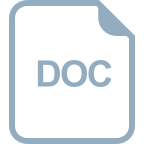








