使用退火法求解bayg29.tsp数据集的最优路径并绘图显示python代码
时间: 2024-02-04 10:04:27 浏览: 30
好的,以下是使用退火算法求解bayg29.tsp数据集最优路径并绘图的Python代码:
```python
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
# 读入数据
data = pd.read_csv('bayg29.csv', header=None, index_col=0)
n = len(data) # 城市数量
# 计算城市之间的距离矩阵
dist = np.zeros((n, n))
for i in range(n):
for j in range(n):
dist[i][j] = np.sqrt((data.iloc[i][0] - data.iloc[j][0]) ** 2 + (data.iloc[i][1] - data.iloc[j][1]) ** 2)
# 定义目标函数
def cost(x):
res = 0
for i in range(n - 1):
res += dist[x[i]][x[i+1]]
res += dist[x[n-1]][x[0]]
return res
# 定义退火算法
def anneal():
x = np.arange(n) # 初始解
best_x = x.copy() # 最优解
T = 1e3 # 初始温度
Tmin = 1e-3 # 温度下限
alpha = 0.99 # 降温速度
while T > Tmin:
for i in range(1000): # 每个温度下进行1000次迭代
# 产生新解
new_x = x.copy()
a = np.random.randint(0, n)
b = np.random.randint(0, n)
while a == b:
b = np.random.randint(0, n)
new_x[a], new_x[b] = new_x[b], new_x[a]
# 判断是否接受新解
delta = cost(new_x) - cost(x)
if delta < 0 or np.exp(-delta / T) > np.random.rand():
x = new_x.copy()
if cost(x) < cost(best_x):
best_x = x.copy()
T *= alpha # 降温
return best_x
# 运行退火算法并绘制结果
best_x = anneal()
print('最优路径长度为:', cost(best_x))
plt.plot(data.iloc[best_x, 0], data.iloc[best_x, 1], '-o')
plt.show()
```
这段代码首先读入了bayg29.tsp数据集,并计算城市之间的距离矩阵。然后定义了目标函数cost和退火算法anneal,最后运行退火算法并绘制结果。运行结果会输出最优路径长度,并绘制最优路径的图像。
阅读全文
相关推荐
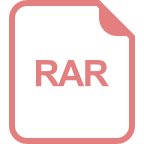
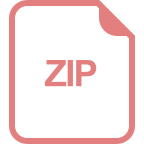


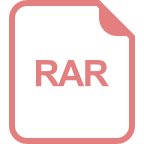


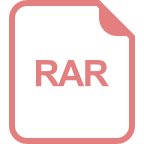
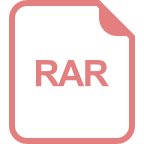
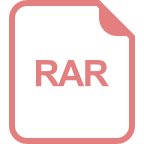
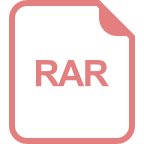
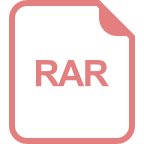
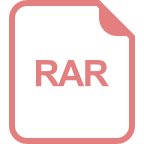
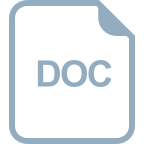
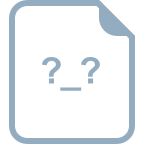
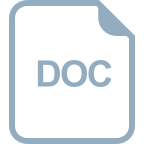
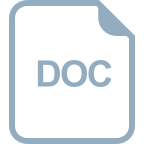
