TypeError: can only concatenate str (not "NoneType") to str
时间: 2023-11-21 14:00:09 浏览: 31
This error occurs when you try to concatenate a string with a None value. In Python, you cannot concatenate a string with None value using the + operator.
For example:
```
string1 = "Hello"
string2 = None
# This will give an error
print(string1 + string2)
```
To fix this error, you can check if the value is None before concatenating it with a string. You can use a conditional statement to check if the value is not None and then proceed with the concatenation.
For example:
```
string1 = "Hello"
string2 = None
if string2 is not None:
print(string1 + string2)
else:
print("string2 is None")
```
This will print "string2 is None" as the value of string2 is None.
相关问题
在scrapy中TypeError: can only concatenate str (not "NoneType") to str报错
在Scrapy中,当出现TypeError: can only concatenate str (not "NoneType") to str报错时,通常是因为在字符串拼接过程中,将None类型的对象与字符串进行了拼接。解决这个问题的方法是先判断对象是否为None,然后再进行拼接操作。
以下是一个解决这个问题的示例代码:
```python
# 判断对象是否为None,如果是则将其转换为空字符串
def check_none(obj):
if obj is None:
return ""
return obj
# 在字符串拼接过程中使用check_none()函数来处理可能为None的对象
str1 = check_none(obj1) + "some string" + check_none(obj2)
```
通过使用check_none()函数,我们可以确保在拼接字符串时不会出现TypeError: can only concatenate str (not "NoneType") to str报错。
解决TypeError: can only concatenate str (not "NoneType") to str问题
TypeError: can only concatenate str (not "NoneType") to str 错误通常发生在试图将 None 类型的值与字符串进行拼接时。这通常表示某个变量的值为 None,而不是字符串。
要解决这个问题,你可以采取以下步骤:
1. 检查代码中涉及到的变量,确保它们都有正确的赋值。特别是,查看被用于拼接的变量是否为 None。
2. 使用条件语句来处理可能为 None 的变量。例如,你可以使用 if 语句来检查变量是否为 None,然后采取相应的处理方式。
下面是一个示例代码,演示了如何处理这个错误:
```
# 假设变量 name 可能为 None
name = None
# 检查 name 是否为 None
if name is not None:
message = "Hello, " + name # 正确地拼接字符串
else:
message = "Hello, stranger" # 处理 name 为 None 的情况
print(message)
```
在这个示例中,我们首先检查 name 是否为 None,然后根据结果进行相应的字符串拼接操作。这样就避免了 TypeError 错误的发生。
请根据你的实际代码情况进行相应的修改和调整。希望对你有所帮助!如果你有更多问题,请继续提问。
相关推荐
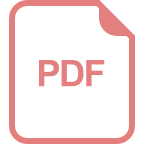
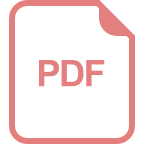
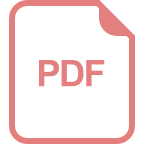












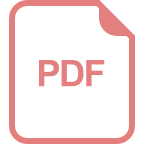