import chardet with open(r'new_comment_content.csv', 'rb') as f: result = chardet.detect(f.read()) encoding = result['encoding'] print("文件编码格式:"+encoding)报错TypeError: can only concatenate str (not "NoneType") to str
时间: 2024-03-06 13:49:04 浏览: 139
这个错误提示是在尝试将一个None类型的值与字符串进行拼接时出现的。根据代码,可能是因为在读取文件时,chardet.detect() 函数返回的 result 中的 encoding 值为 None,导致在字符串拼接时出现错误。可以在使用 result['encoding'] 前,先加一个判断语句,判断 encoding 是否为 None,如果是则手动指定一个默认值。例如:
```
import chardet
with open(r'new_comment_content.csv', 'rb') as f:
result = chardet.detect(f.read())
encoding = result['encoding'] if result['encoding'] is not None else 'utf-8'
print("文件编码格式:"+encoding)
```
这样可以避免出现 TypeError: can only concatenate str (not "NoneType") to str 错误。
相关问题
import chardet with open("/Users/zhoulexin/Downloads/train.csv", 'rb') as f: result = chardet.detect(f.read()) df = pd.read_csv("/Users/zhoulexin/Downloads/train.csv", encoding=result['encoding'])报错为UnicodeDecodeError: 'gb2312' codec can't decode byte 0xe9 in position 63596: illegal multibyte sequence怎么解决
这个错误通常表示文件的编码与你指定的编码不同。可能的解决方法是尝试使用其他编码类型打开文件,或者使用更通用的编码类型(如UTF-8)。你可以尝试下面的代码:
```
import chardet
import pandas as pd
with open("/Users/zhoulexin/Downloads/train.csv", 'rb') as f:
result = chardet.detect(f.read())
df = pd.read_csv("/Users/zhoulexin/Downloads/train.csv", encoding=result['encoding'] or 'utf-8')
```
在这个代码块中,我们使用chardet模块来检测文件的编码类型。如果检测到的编码不是GB2312,则使用UTF-8。这个方法可能不是完全准确的,但是可以尝试。如果还是有问题,你可以再试试其他编码类型,例如GBK等。
import pandas as pd import os import chardet path1 = 'D:/jupyter_load/guobu/data_list/' csv_files = [file for file in os.listdir(path1) if file.endswith('.csv')] #获取文件夹中的所有以.csv为后缀的文件 #创建一个空的DataFrame用于存储合并后的数据 merge_data = pd.DataFrame() #for循环遍历每一个csv文件并进行合并 for file in csv_files: file_path = os.path.join(path1,file) #构建文件路径,path后面会加上xxx.csv文件 with open('file_path', 'rb') as f: result = chardet.detect(f.read()) encoding = result['encoding'] df = pd.read_csv(path,encoding='encoding') merged_data = merged_data.append(df, ignore_index=True) print(merge_data) 为什么会报错,哪里错了?
在你的代码中,有几个地方可能会出错:
1. `path` 变量未定义:在 `pd.read_csv()` 函数中,你使用了一个未定义的变量 `path`。你可能想使用的是 `file_path`。请将 `pd.read_csv(path, encoding='encoding')` 改为 `pd.read_csv(file_path, encoding=encoding)`。
2. `merged_data` 变量未定义:在每次循环迭代时,你将读取的数据存储在变量 `df` 中,但是你试图将其追加到一个未定义的变量 `merged_data` 中。请将 `merged_data` 改为 `merge_data`。
3. 打开文件时使用了错误的文件路径:在使用 `chardet` 检测文件编码时,你使用了字符串 `'file_path'`,而不是变量 `file_path`。请将 `with open('file_path', 'rb') as f:` 改为 `with open(file_path, 'rb') as f:`。
修正后的代码如下:
```python
import pandas as pd
import os
import chardet
path1 = 'D:/jupyter_load/guobu/data_list/'
csv_files = [file for file in os.listdir(path1) if file.endswith('.csv')] #获取文件夹中的所有以.csv为后缀的文件
#创建一个空的DataFrame用于存储合并后的数据
merge_data = pd.DataFrame()
#for循环遍历每一个csv文件并进行合并
for file in csv_files:
file_path = os.path.join(path1,file) #构建文件路径,path后面会加上xxx.csv文件
with open(file_path, 'rb') as f:
result = chardet.detect(f.read())
encoding = result['encoding']
df = pd.read_csv(file_path, encoding=encoding)
merge_data = merge_data.append(df, ignore_index=True)
print(merge_data)
```
请注意,我进行了上述修正,但仍然建议你仔细检查代码,确保路径和文件名的正确性,以及确保文件夹中的所有文件都是有效的 CSV 文件。
阅读全文
相关推荐
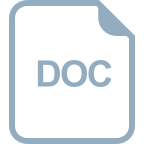
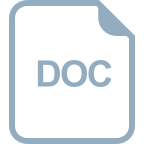
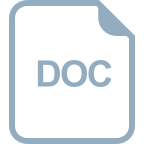














