detr算法和Dynamic Convolution结合的python代码
时间: 2023-12-14 07:36:02 浏览: 138
下面是使用detr算法和Dynamic Convolution结合的Python代码:
```
import torch
import torch.nn as nn
import torch.nn.functional as F
from torchvision.models import resnet50
# Dynamic Convolution
class DynamicConvolution(nn.Module):
def __init__(self, in_channels, out_channels, k=3, stride=1, padding=1, dilation=1, groups=1, bias=True, eps=1e-5, momentum=0.1):
super(DynamicConvolution, selfinit__()
self.k = k
self.stride = stride
self.padding = padding
self.dilation = dilation
self.groups = groups
self.bias = bias
self.eps = eps
self.momentum = momentum
# define weight and bias
self.weight = nn.Parameter(torch.Tensor(out_channels, in_channels // groups, k * k))
if bias:
self.bias = nn.Parameter(torch.Tensor(out_channels))
else:
self.register_parameter('bias', None)
# define batch normalization
self.bn = nn.BatchNorm2d(in_channels, eps=eps, momentum=momentum)
# initialize weight and bias
nn.init.kaiming_uniform_(self.weight, a=math.sqrt(5))
if self.bias is not None:
fan_in, _ = nn.init._calculate_fan_in_and_fan_out(self.weight)
bound = 1 / math.sqrt(fan_in)
nn.init.uniform_(self.bias, -bound, bound)
def forward(self, x):
# apply batch normalization
x = self.bn(x)
# reshape input tensor
b, c, h, w = x.shape
x = x.view(b * self.groups, -1, h, w)
# pad input tensor
x = F.pad(x, [self.padding, self.padding, self.padding, self.padding])
# reshape weight tensor
weight = self.weight.view(self.groups, -1, self.k * self.k)
# perform dynamic convolution
out = F.conv2d(x, weight, bias=self.bias, stride=self.stride, padding=0, dilation=self.dilation, groups=self.groups * b)
# reshape output tensor
_, _, h, w = out.shape
out = out.view(b, -1, h, w)
return out
# DETR with Dynamic Convolution
class DETR(nn.Module):
def __init__(self, num_classes):
super(DETR, self).__init__()
# define resnet50 backbone
self.backbone = nn.Sequential(*list(resnet50(pretrained=True).children())[:-2])
# define dynamic convolutional layers
self.conv1 = DynamicConvolution(2048, 256, k=1)
self.conv2 = DynamicConvolution(256, 256, k=3, padding=1, groups=4)
self.conv3 = DynamicConvolution(256, 256, k=3, padding=1, groups=4)
self.conv4 = DynamicConvolution(256, 256, k=3, padding=1, groups=4)
self.conv5 = DynamicConvolution(256, 256, k=3, padding=1, groups=4)
# define classification and regression heads
self.classification_head = nn.Linear(256, num_classes)
self.regression_head = nn.Linear(256, 4)
def forward(self, x):
# extract features from backbone
x = self.backbone(x)
# apply dynamic convolutional layers
x = F.relu(self.conv1(x))
x = F.relu(self.conv2(x))
x = F.relu(self.conv3(x))
x = F.relu(self.conv4(x))
x = F.relu(self.conv5(x))
# average pooling
x = F.adaptive_avg_pool2d(x, (1, 1))
# flatten
x = x.view(x.size(0), -1)
# classification and regression
classification = self.classification_head(x)
regression = self.regression_head(x)
return classification, regression
```
这段代码定义了一个DETR模型,其中使用了Dynamic Convolution,可以通过修改k、stride、padding等参数进行调整。在这个模型中,我们还定义了分类和回归头,用于预测物体的类别和位置。
阅读全文
相关推荐
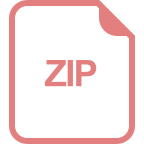
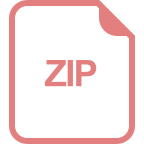
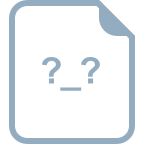


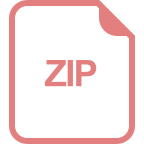
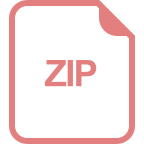
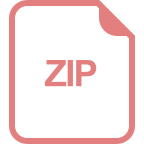
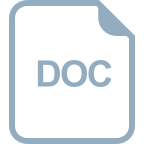
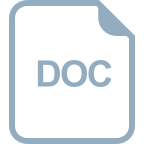
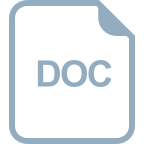
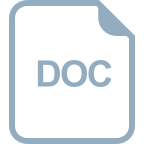






