检测excel文件的名字在.c文件是否存在,并输出结果
时间: 2024-05-13 18:20:56 浏览: 23
这个任务可以通过以下步骤实现:
1. 读取.c文件名和excel文件名。
2. 检查excel文件名是否在.c文件中存在。
3. 如果存在,输出“文件已存在”;如果不存在,输出“文件不存在”。
下面是一个Python示例代码,用于检测excel文件名是否在.c文件中存在:
```python
import os
# 读取.c文件名和excel文件名
c_file = input("请输入.c文件名:")
excel_file = input("请输入excel文件名:")
# 检查excel文件名是否在.c文件中存在
if os.path.exists(c_file):
with open(c_file, "r") as f:
content = f.read()
if excel_file in content:
print("文件已存在")
else:
print("文件不存在")
else:
print(".c文件不存在")
```
这个代码使用了Python内置的os模块,它提供了许多有用的操作系统交互功能,包括文件和目录操作。在这个代码中,我们使用了os.path.exists()函数来检查.c文件是否存在,使用open()函数打开.c文件并读取其中的内容,然后使用in关键字来检查excel文件名是否在其中。最后,我们使用print()函数输出结果。
相关问题
检测excel文件中这一列的所有的变量名在.c文件中是否存在,并输出结果
这个任务可以通过编写一个Python脚本来完成。以下是可能的解决方案:
1. 首先,需要安装必要的Python库:pandas、os和re。可以使用pip命令来安装它们。
2. 加载Excel文件并提取需要检查的列。可以使用pandas库来完成这个任务。
3. 确定需要检查的.c文件的目录,并使用os库中的方法列出所有文件。
4. 对于每个.c文件,读取其内容并使用re库中的正则表达式搜索变量名。
5. 输出所有未找到的变量名。
下面是可能的Python脚本,根据具体情况可以进行修改和优化:
```python
import pandas as pd
import os
import re
# 加载Excel文件
df = pd.read_excel('file.xlsx', sheet_name='Sheet1')
# 提取需要检查的列
col_name = 'Variable Name'
var_names = df[col_name].tolist()
# 确定需要检查的.c文件的目录
dir_path = '/path/to/c/files'
files = [f for f in os.listdir(dir_path) if f.endswith('.c')]
# 遍历所有.c文件并搜索变量名
not_found = []
for f in files:
with open(os.path.join(dir_path, f), 'r') as file:
content = file.read()
for var in var_names:
if not re.search(r'\b{}\b'.format(var), content):
not_found.append(var)
# 输出所有未找到的变量名
print('Variables not found:')
print('\n'.join(not_found))
```
注意,这个脚本假设变量名在代码中是以单词的形式出现的,即被空格、符号或行结束符包围。如果变量名出现在注释或字符串中,则这个脚本将不会找到它们。因此,需要根据具体情况进行修改和测试。
检测excel文件中这一列的所有的变量名在.c文件中是否存在,并输出excel
文件中每个变量名对应的存在情况。
这个任务可以通过以下步骤完成:
1. 从excel文件中读取需要检测的变量名所在的列,保存到一个列表中。
2. 读取.c文件内容,并将其中的变量名保存到一个字典中,字典的键为变量名,值为True。
3. 遍历步骤1中保存的变量名列表,对于每个变量名,在字典中查找是否存在对应的键。如果存在,将该变量名在excel文件中的行号和存在情况(存在/不存在)保存到一个列表中。
4. 将步骤3中保存的结果输出到excel文件中,作为新的一列。
以下是一个示例代码,用于实现这个任务:
```python
import openpyxl
# 读取excel文件中需要检测的变量名所在的列
def read_excel_column(filename, sheetname, column):
wb = openpyxl.load_workbook(filename)
sheet = wb[sheetname]
values = []
for cell in sheet[column]:
values.append(cell.value)
return values
# 读取.c文件内容,并将其中的变量名保存到一个字典中
def read_c_file(filename):
variables = {}
with open(filename, 'r') as f:
for line in f:
words = line.split()
for word in words:
if word.isalpha():
variables[word] = True
return variables
# 检测变量名在.c文件中是否存在,并保存结果到列表中
def check_variables(variables, c_variables):
result = []
for i, var in enumerate(variables):
if var in c_variables:
result.append([i+1, '存在'])
else:
result.append([i+1, '不存在'])
return result
# 将检测结果保存到excel文件中
def save_excel(filename, sheetname, column, result):
wb = openpyxl.load_workbook(filename)
sheet = wb[sheetname]
for i, value in enumerate(result):
sheet[column+str(i+1)] = value[1]
wb.save(filename)
# 测试
if __name__ == '__main__':
# 读取excel文件中需要检测的变量名
variables = read_excel_column('test.xlsx', 'Sheet1', 'A')
# 读取.c文件中的变量名
c_variables = read_c_file('test.c')
# 检测变量名在.c文件中是否存在
result = check_variables(variables, c_variables)
# 将检测结果保存到excel文件中
save_excel('test.xlsx', 'Sheet1', 'B', result)
```
在运行以上代码之前,请确保已经安装了openpyxl模块,可以通过以下命令安装:
```
pip install openpyxl
```
相关推荐
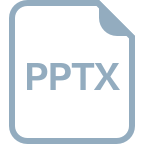
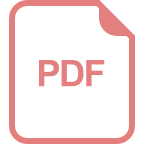
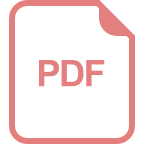












