爬取豆瓣读书top250保存到mysql完整代码
时间: 2023-07-15 20:12:35 浏览: 100
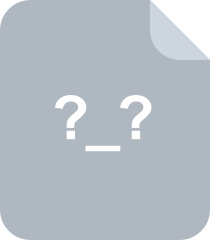
Python爬取豆瓣250数据保存到MySQL或者excel代码
以下是爬取豆瓣读书top250并将数据保存到MySQL数据库的完整代码:
```python
import requests
from bs4 import BeautifulSoup
import pymysql
# 连接MySQL数据库
db = pymysql.connect(host='localhost', user='root', password='password', database='douban', port=3306)
cursor = db.cursor()
# 创建表格
create_table_sql = """
CREATE TABLE IF NOT EXISTS `book` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`title` varchar(255) DEFAULT NULL,
`author` varchar(255) DEFAULT NULL,
`score` float DEFAULT NULL,
`comment_num` int(11) DEFAULT NULL,
`url` varchar(255) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
"""
cursor.execute(create_table_sql)
# 爬取豆瓣读书top250
for i in range(0, 250, 25):
url = f'https://book.douban.com/top250?start={i}'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
book_list = soup.find('div', class_='article').find_all('table')
# 解析每一本书的信息并保存到数据库
for book in book_list:
title = book.find('div', class_='pl2').find('a')['title']
author = book.find('p', class_='pl').get_text()
score = book.find('span', class_='rating_nums').get_text()
comment_num = book.find('span', class_='pl').get_text().split()[1].strip('()')
url = book.find('div', class_='pl2').find('a')['href']
# 插入数据到MySQL数据库
insert_sql = f"INSERT INTO `book` (`title`, `author`, `score`, `comment_num`, `url`) VALUES ('{title}', '{author}', {score}, {comment_num}, '{url}')"
try:
cursor.execute(insert_sql)
db.commit()
print(f"{title} 保存成功")
except Exception as e:
db.rollback()
print(f"{title} 保存失败,错误信息:{e}")
# 关闭数据库连接
db.close()
```
注意:
1. 需要将代码中的数据库连接信息改为自己的实际信息。
2. 该代码使用的是豆瓣读书top250的网页结构,如果网页结构有改变,需要相应地修改代码。
3. 插入数据到MySQL数据库时使用的是字符串拼接方式,存在SQL注入的风险,建议使用参数化查询来避免此风险。
阅读全文
相关推荐
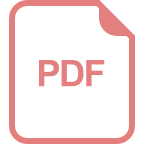

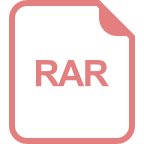
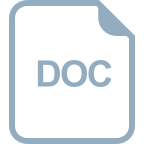




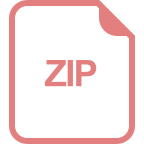
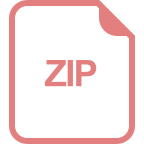
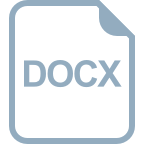
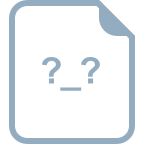
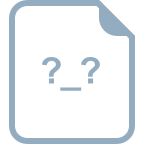
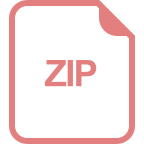


