用C语言编写一个stm32函数,用两个io采集脉冲编码开关输出,判定开关位置
时间: 2023-05-31 10:05:05 浏览: 54
以下是一个基本的示例代码,用于读取两个IO口的脉冲编码开关输入,并确定其位置。
```c
#include "stm32f10x.h"
#define ENCODER_A_PORT GPIOA
#define ENCODER_A_PIN GPIO_Pin_0
#define ENCODER_B_PORT GPIOA
#define ENCODER_B_PIN GPIO_Pin_1
uint8_t encoder_state = 0;
int16_t encoder_position = 0;
void encoder_init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = ENCODER_A_PIN | ENCODER_B_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
encoder_state = (GPIO_ReadInputData(ENCODER_A_PORT) << 1) | GPIO_ReadInputData(ENCODER_B_PORT);
}
void encoder_update(void)
{
static uint8_t old_encoder_state = 0;
uint8_t new_encoder_state = (GPIO_ReadInputData(ENCODER_A_PORT) << 1) | GPIO_ReadInputData(ENCODER_B_PORT);
if (new_encoder_state != old_encoder_state)
{
if ((old_encoder_state == 0 && new_encoder_state == 2) ||
(old_encoder_state == 1 && new_encoder_state == 3) ||
(old_encoder_state == 2 && new_encoder_state == 0) ||
(old_encoder_state == 3 && new_encoder_state == 1))
{
encoder_position--;
}
else if ((old_encoder_state == 0 && new_encoder_state == 1) ||
(old_encoder_state == 1 && new_encoder_state == 0) ||
(old_encoder_state == 2 && new_encoder_state == 3) ||
(old_encoder_state == 3 && new_encoder_state == 2))
{
encoder_position++;
}
old_encoder_state = new_encoder_state;
}
}
int main(void)
{
encoder_init();
while (1)
{
encoder_update();
// Do something with encoder_position
}
}
```
在代码中,我们首先定义了两个IO口分别用于连接编码开关的A和B信号。在`encoder_init`函数中,我们初始化了这两个IO口,并读取了它们的初始状态。然后,在`encoder_update`函数中,我们检测A和B信号的状态变化,并根据变化来更新编码器的位置。最后,在主循环中,我们不断调用`encoder_update`函数,并在需要时使用`encoder_position`变量来判定开关位置。
相关推荐
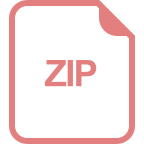
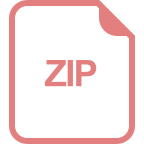














