javaweb登陆页面源代码
时间: 2023-09-13 17:09:25 浏览: 116
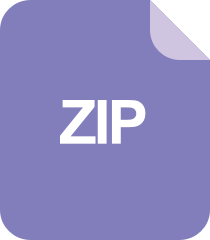
java源码 仿京东JavaWeb项目源代码.zip
以下是一个简单的JavaWeb登陆页面的源代码示例:
1. login.jsp 页面
```html
<!DOCTYPE html>
<html>
<head>
<title>Login</title>
</head>
<body>
<h2>Login</h2>
<form action="login" method="post">
<label for="username">Username:</label>
<input type="text" id="username" name="username"><br><br>
<label for="password">Password:</label>
<input type="password" id="password" name="password"><br><br>
<input type="submit" value="Login">
</form>
</body>
</html>
```
2. LoginServlet.java Servlet
```java
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
public class LoginServlet extends HttpServlet {
// 用户名和密码,这里用硬编码方式存储,实际应该是从数据库或文件读取
private String username = "admin";
private String password = "123456";
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String username = request.getParameter("username");
String password = request.getParameter("password");
// 判断用户名和密码是否正确
if (username.equals(this.username) && password.equals(this.password)) {
// 创建 HttpSession 对象并将用户名存储到 Session 对象中
HttpSession session = request.getSession();
session.setAttribute("username", username);
// 重定向到首页
response.sendRedirect(request.getContextPath() + "/index.jsp");
} else {
// 登录失败,重新返回到登录界面
response.setContentType("text/html;charset=utf-8");
PrintWriter out = response.getWriter();
out.println("<html>");
out.println("<head><title>登录失败</title></head>");
out.println("<body>");
out.println("<script>alert('Login failed. Please try again.');</script>");
out.println("<a href='login.jsp'>Back to Login</a>");
out.println("</body>");
out.println("</html>");
}
}
}
```
在这个例子中,我们通过判断用户名和密码是否正确来实现登录验证。如果用户名和密码正确,我们将创建 HttpSession 对象并将用户名存储到 Session 对象中,然后将用户重定向到首页。如果登录失败,我们将在页面上显示一个消息并将用户重定向回登录页面。
注意,此处的用户名和密码是硬编码,实际应该从数据库或文件中读取。另外,这个例子没有进行任何安全性检查,实际应用中需要考虑加强安全性。
阅读全文
相关推荐
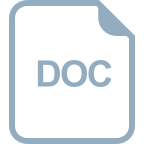
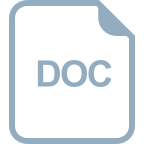
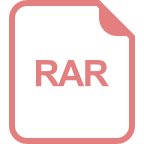
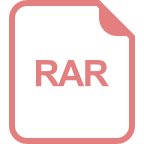
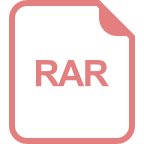
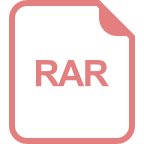
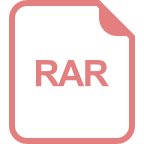




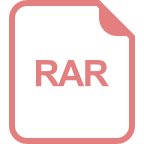
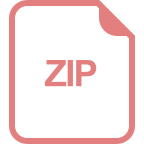
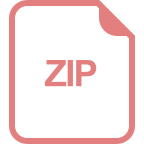
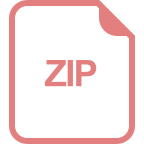
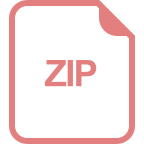
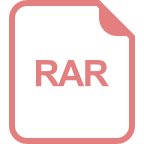
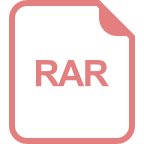