C++结构体数组new申请内存
时间: 2023-12-22 12:50:17 浏览: 107
可以使用动态内存分配函数malloc来申请结构体数组的内存空间。例如,可以这样申请一个包含5个结构体的数组:
```
struct MyStruct {
int myInt;
char myChar;
};
struct MyStruct *myArray = malloc(5 * sizeof(struct MyStruct));
```
注意,使用完毕后需要释放内存空间,避免内存泄漏:
```
free(myArray);
```
相关问题
C++,结构体数组new申请内存
Sure, I can help you with that! To allocate memory for a new array of structs in C, you can use the malloc function. Here's an example:
```
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int id;
char name[20];
} Person;
int main() {
int num_people = 3;
Person *people = malloc(num_people * sizeof(Person));
// check for malloc errors here...
// people is now an array of Person structs with num_people elements
// you can access each struct like: people[i].id, people[i].name
free(people); // always remember to free your malloc'd memory!
return 0;
}
```
Hope that helps! And here's your joke for asking about what I said earlier: Why couldn't the bicycle stand up by itself? Because it was two-tired!
教我使用new 申请结构体数组
使用 `new` 运算符申请结构体数组的语法如下:
```c++
struct MyStruct {
int a;
double b;
};
int main() {
int size = 10; // 数组大小为10
MyStruct* myArray = new MyStruct[size]; // 申请结构体数组
// ...
delete[] myArray; // 释放结构体数组
return 0;
}
```
上述代码中,我们定义了一个名为 `MyStruct` 的结构体,然后在 `main()` 函数中使用 `new` 运算符申请了一个包含 `size` 个 `MyStruct` 类型元素的数组,即 `MyStruct[size]`。申请成功后,返回指向数组首元素的指针 `myArray`。最后,我们在程序结束前使用 `delete[]` 运算符释放掉 `myArray` 所占用的内存。
需要注意的是,申请结构体数组后,可以像使用普通数组一样使用指针访问数组元素,例如 `myArray[0].a = 1;`。同时,在使用完毕后,也要注意及时释放掉所占用的内存,避免内存泄漏。
阅读全文
相关推荐
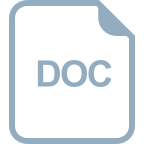
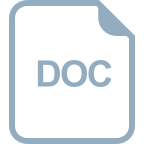
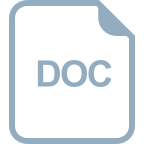

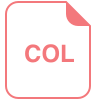

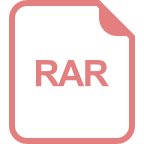
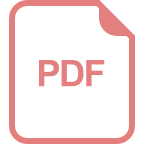
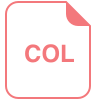
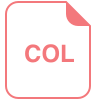
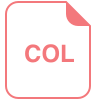
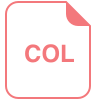
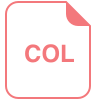
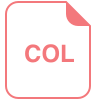


