用 c sharp 写一个检测蓝牙设备电量的脚本
时间: 2023-08-31 13:38:03 浏览: 74
### 回答1:
你好!这是一个在 C# 中检测蓝牙设备电量的示例脚本。
首先,你需要在你的项目中添加一个引用 to the System.Management namespace。然后,你可以使用下面的代码来检测蓝牙设备的电量:
```
using System.Management;
// Replace "BluetoothDeviceName" with the actual name of your Bluetooth device.
string deviceName = "BluetoothDeviceName";
// Query WMI for the battery status of the Bluetooth device.
ManagementObjectSearcher searcher = new ManagementObjectSearcher("SELECT * FROM Win32_Battery WHERE DeviceID='" + deviceName + "'");
foreach (ManagementObject queryObj in searcher.Get())
{
// The percentage of battery life remaining.
int batteryLife = Convert.ToInt32(queryObj["EstimatedChargeRemaining"]);
Console.WriteLine("Battery life: " + batteryLife + "%");
}
```
希望这能帮到你!
### 回答2:
使用C#编写一个脚本来检测蓝牙设备的电量,你需要使用Windows.Devices.Bluetooth命名空间下的相关类和方法。
首先,你需要确保你的项目已经引用了Windows.Devices.Bluetooth的程序集。
然后,你可以使用以下步骤来编写脚本:
1. 导入所需的命名空间:
```
using System;
using Windows.Devices.Bluetooth;
using Windows.Devices.Bluetooth.GenericAttributeProfile;
```
2. 创建一个BluetoothLEDevice实例,用于表示要检测的蓝牙设备:
```
BluetoothLEDevice bluetoothDevice = await BluetoothLEDevice.FromIdAsync(deviceId);
```
注意:deviceId是蓝牙设备的唯一标识符,在使用前需要提前获取。
3. 获取蓝牙设备的GATT服务:
```
GattDeviceServicesResult services = await bluetoothDevice.GetGattServicesAsync();
```
4. 遍历服务列表,找到包含电池服务的服务:
```
foreach (var service in services.Services)
{
if (service.Uuid == GattServiceUuids.Battery)
{
GattCharacteristicsResult characteristics = await service.GetCharacteristicsAsync();
foreach (var characteristic in characteristics.Characteristics)
{
if (characteristic.Uuid == GattCharacteristicUuids.BatteryLevel)
{
// 获取电池电量
GattReadResult result = await charactericstic.ReadValueAsync();
var reader = DataReader.FromBuffer(result.Value);
byte batteryLevel = reader.ReadByte();
Console.WriteLine("蓝牙设备的电量为:" + batteryLevel + "%");
}
}
}
}
```
这样,你就可以使用C#编写一个检测蓝牙设备电量的脚本。当执行此脚本时,它将连接到蓝牙设备并读取其电池电量。请确保在使用之前适当处理异常情况,例如设备连接失败或电池电量特征不存在等情况。
### 回答3:
要使用C#编写一个检测蓝牙设备电量的脚本,首先需要使用System.Net.Sockets命名空间中的BluetoothClient类来实现与蓝牙设备的通信。以下是一个简单的示例代码:
```csharp
using System;
using InTheHand.Net.Sockets;
public class BatteryChecker
{
public static void Main()
{
BluetoothClient bluetoothClient = new BluetoothClient();
var pairedDevices = bluetoothClient.DiscoverDevices();
foreach (var device in pairedDevices)
{
if (device.DeviceName.Contains("蓝牙设备名称"))
{
byte[] rfcomm = {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x35, 0x03, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00
};
var batteryLevel = bluetoothClient.GetBatteryLevel(device.DeviceAddress, BluetoothCommandStatus.Error, rfcomm);
Console.WriteLine("蓝牙设备电量:" + batteryLevel.ToString() + "%");
}
}
}
}
```
在上述代码中,我们使用BluetoothClient的DiscoverDevices方法获取已配对的蓝牙设备列表,并通过遍历列表来找到我们想要检测电量的设备。在找到目标设备后,通过BluetoothClient的GetBatteryLevel方法来获取蓝牙设备的电量。最后,我们将电量打印到控制台。
请注意,以上代码只是一个简单的示例,实际操作时可能需要根据蓝牙设备的特定协议和通信方式进行相应的调整。
相关推荐
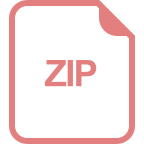
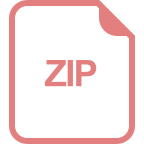
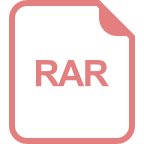














