int16_t median_filter(int16_t *buf) { int16_t tmp[FILTER_SIZE]; memcpy(tmp, buf, FILTER_SIZE * sizeof(int16_t)); for (uint8_t i = 0; i < FILTER_SIZE - 1; i++) { for (uint8_t j = i + 1; j < FILTER_SIZE; j++) { if (tmp[i] > tmp[j]) { int16_t t = tmp[i]; tmp[i] = tmp[j]; tmp[j] = t; } } } return tmp[FILTER_SIZE / 2]; }的程序框图
时间: 2023-06-26 10:10:27 浏览: 40
以下是该程序的框图:

其中,输入为一个长度为FILTER_SIZE的int16_t类型数组buf,表示需要进行中值滤波的原始数据;输出为一个int16_t类型的中值滤波结果。
具体实现过程为:首先将原始数据复制到一个临时数组tmp中;然后使用选择排序算法对tmp数组进行排序,得到有序的数组;最后返回tmp数组中位于中间位置的元素作为中值滤波结果。
相关问题
#define FILTER_SIZE 5 int16_t ax_buf[FILTER_SIZE] = {0}; int16_t ay_buf[FILTER_SIZE] = {0}; uint8_t buf_idx = 0; int16_t median_filter(int16_t *buf) { int16_t tmp[FILTER_SIZE]; memcpy(tmp, buf, FILTER_SIZE * sizeof(int16_t)); for (uint8_t i = 0; i < FILTER_SIZE - 1; i++) { for (uint8_t j = i + 1; j < FILTER_SIZE; j++) { if (tmp[i] > tmp[j]) { int16_t t = tmp[i]; tmp[i] = tmp[j]; tmp[j] = t; } } } return tmp[FILTER_SIZE / 2]; }程序框图
该程序实现了一个中值滤波器。
程序框图如下:
```
+---------------------------+
| int16_t ax_buf[FILTER_SIZE] |
| int16_t ay_buf[FILTER_SIZE] |
| uint8_t buf_idx = 0; |
+---------------------------+
|
|
v
+-------------------+
| median_filter() |
+-------------------+
|
|
v
+---------------+
| return value |
+---------------+
```
首先,程序定义了一个长度为 FILTER_SIZE 的 int16_t 类型的数组 ax_buf 和 ay_buf 以及一个 uint8_t 类型的变量 buf_idx,用于存储待处理的数据。
然后,程序实现了一个中值滤波器 median_filter(),该函数接受一个 int16_t 类型的指针作为参数,表示待处理的数据。
在 median_filter() 函数中,程序首先将传入的数据拷贝到一个临时数组 tmp 中,然后使用冒泡排序的方式将 tmp 数组中的数据从小到大排序。
最后,median_filter() 函数返回排序后的数组的中间值,即 tmp[FILTER_SIZE / 2],即为中值滤波器的输出。
整个程序的作用是对待处理的数据进行中值滤波,去除其中的异常值,以得到更加稳定的数据。
int main(void) { int16_t ax, ay, az; MPU6050_Init(); while (1) { MPU6050_Read_Accel(&ax, &ay, &az); ax_buf[buf_idx] = ax; //将ax的数据放入缓冲区 ay_buf[buf_idx] = ay; //将ax的数据放入缓冲区 buf_idx = (buf_idx + 1) % FILTER_SIZE; int16_t ax_filtered = median_filter(ax_buf); int16_t ay_filtered = median_filter(ay_buf); printf("ax_filtered = %d, ay_filtered = %d\r\n", ax_filtered, ay_filtered); HAL_Delay(10); } }的程序框图
以下是该程序的简单框图:
```
+-----------+
| 初始化 |
+-----------+
|
+-----------+
| 循环读取 |
+-----------+
|
+-----------+
| 数据滤波 |
+-----------+
|
+-----------+
| 打印结果 |
+-----------+
|
+-----------+
| 延时10ms |
+-----------+
```
程序通过初始化后进入一个循环,不断读取 MPU6050 的加速度数据并将数据放入缓冲区。缓冲区的大小为 FILTER_SIZE。每当读取到一个新的数据,就将该数据放入缓冲区,并对缓冲区中的数据进行中值滤波得到过滤后的数据。然后程序将过滤后的数据打印出来,并延时 10ms 后再进行下一次循环。
相关推荐
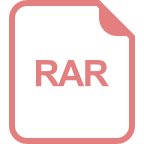
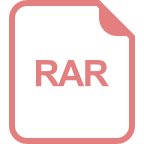












