#include <iostream>#include <chrono>#include <thread>void increaseNumPerSecond(int& num) { while (true) { num++; std::this_thread::sleep_for(std::chrono::seconds(1)); }}int main() { int num = 0; std::thread t(increaseNumPerSecond, std::ref(num)); while (true) { std::cout << "Current number: " << num << std::endl; std::this_thread::sleep_for(std::chrono::milliseconds(500)); } t.join(); return 0;}
时间: 2023-07-16 07:12:31 浏览: 55
这是一个 C++ 程序,它创建了一个线程来每秒钟增加一个整数变量 num 的值,同时在主线程中每 500 毫秒输出 num 的当前值。程序的主要部分如下所示:
```c++
void increaseNumPerSecond(int& num) {
while (true) {
num++;
std::this_thread::sleep_for(std::chrono::seconds(1));
}
}
int main() {
int num = 0;
std::thread t(increaseNumPerSecond, std::ref(num));
while (true) {
std::cout << "Current number: " << num << std::endl;
std::this_thread::sleep_for(std::chrono::milliseconds(500));
}
t.join();
return 0;
}
```
首先定义了一个名为 `increaseNumPerSecond` 的函数,它接受一个整数引用作为参数,然后在一个无限循环中每秒钟将该整数加 1,并通过 `std::this_thread::sleep_for` 函数暂停 1 秒钟。
接下来是 `main` 函数,它声明了一个整数变量 `num`,并创建了一个新的线程 `t`,该线程将调用 `increaseNumPerSecond` 函数并传递 `num` 的引用作为参数。然后,在一个无限循环中,主线程每 500 毫秒输出 `num` 的当前值,并通过 `std::this_thread::sleep_for` 函数暂停 500 毫秒。最后,调用 `t.join()` 等待线程 `t` 结束,并返回 0。
这个程序可以用来演示如何使用 C++11 中的线程库创建一个简单的多线程程序。
相关推荐
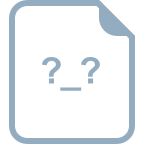














