数据结构头插法建立单链表
时间: 2023-05-28 12:06:05 浏览: 83
头插法是一种向链表头部插入新节点的方法,它可以快速地建立单链表。下面是头插法建立单链表的步骤:
1. 定义链表的节点结构体,包含数据域和指针域。
struct ListNode {
int val;
ListNode *next;
ListNode(int x) : val(x), next(NULL) {}
};
2. 创建一个头节点,并将头节点的指针域初始化为 NULL。
ListNode *head = new ListNode(0);
head->next = NULL;
3. 从标准输入读入数据,每次读入一个数,就创建一个新节点,将读入的数存入新节点的数据域,并将新节点插入到链表的头部。
int x;
while (cin >> x) {
ListNode *node = new ListNode(x);
node->next = head->next;
head->next = node;
}
4. 输出链表的值。
ListNode *p = head->next;
while (p != NULL) {
cout << p->val << " ";
p = p->next;
}
完整代码如下:
#include <iostream>
using namespace std;
struct ListNode {
int val;
ListNode *next;
ListNode(int x) : val(x), next(NULL) {}
};
int main() {
ListNode *head = new ListNode(0);
head->next = NULL;
int x;
while (cin >> x) {
ListNode *node = new ListNode(x);
node->next = head->next;
head->next = node;
}
ListNode *p = head->next;
while (p != NULL) {
cout << p->val << " ";
p = p->next;
}
return 0;
}
阅读全文
相关推荐
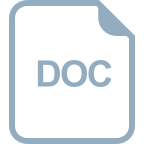
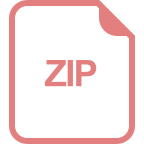
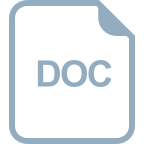
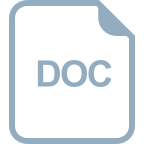
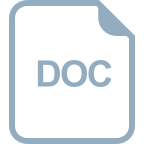
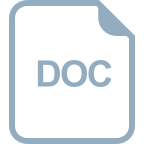
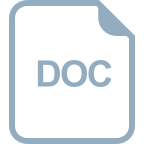






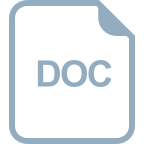
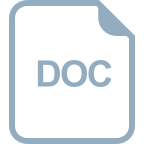
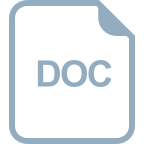