用MATLAB编程,使用蚁群算法解决旅行商问题
时间: 2023-06-12 08:08:20 浏览: 61
旅行商问题是一个经典的组合优化问题,它的目标是找到一条路线,使得从起点出发,经过所有的城市后,最终回到起点的总路程最短。蚁群算法是一种基于自然界中蚂蚁觅食行为而发展起来的一种随机化搜索算法,可以用来解决旅行商问题。
下面是使用MATLAB编程实现蚁群算法解决旅行商问题的步骤:
1. 初始化问题参数和蚁群算法参数,包括城市数量、蚂蚁数量、迭代次数、信息素增量和挥发系数等。
2. 随机生成一组初始解,即蚂蚁的路径。
3. 对于每一只蚂蚁,根据信息素和距离计算出每个城市的选择概率,并根据概率选择下一个城市。
4. 更新信息素,包括信息素增量和信息素挥发。
5. 计算所有蚂蚁的路径长度,取最短路径作为当前最优解。
6. 重复步骤3-5,直到达到迭代次数。
7. 输出最优解。
下面是一个简单的MATLAB代码实现:
```matlab
% 初始化参数
num_cities = 10; % 城市数量
num_ants = 20; % 蚂蚁数量
num_iterations = 100; % 迭代次数
alpha = 1; % 信息素重要程度因子
beta = 2; % 距离重要程度因子
rho = 0.5; % 信息素挥发因子
Q = 100; % 信息素增量常数
% 随机生成城市坐标
cities = 100 * rand(num_cities, 2);
% 计算城市之间的距离
distances = pdist2(cities, cities);
% 初始化信息素矩阵
pheromones = ones(num_cities, num_cities);
% 开始迭代
for iter = 1:num_iterations
% 初始化蚂蚁的路径
paths = zeros(num_ants, num_cities);
for ant = 1:num_ants
% 选择起点城市
current_city = randi(num_cities);
path = current_city;
% 选择下一个城市
for i = 2:num_cities
% 计算城市选择概率
prob = pheromones(current_city,:) .^ alpha .* (1 ./ distances(current_city,:)) .^ beta;
prob(path) = 0;
prob = prob / sum(prob);
% 选择下一个城市
next_city = randsample(num_cities, 1, true, prob);
path(i) = next_city;
current_city = next_city;
end
% 更新路径
paths(ant,:) = path;
end
% 计算路径长度
lengths = zeros(num_ants, 1);
for ant = 1:num_ants
path = paths(ant,:);
length = sum(distances(sub2ind([num_cities,num_cities],path,[path(2:end),path(1)])));
lengths(ant) = length;
end
% 更新信息素
delta_pheromones = zeros(num_cities, num_cities);
for ant = 1:num_ants
path = paths(ant,:);
for i = 1:num_cities
delta_pheromones(path(i), path(mod(i,num_cities)+1)) = delta_pheromones(path(i), path(mod(i,num_cities)+1)) + Q / lengths(ant);
end
end
pheromones = (1-rho) * pheromones + delta_pheromones;
% 输出结果
[min_length, min_index] = min(lengths);
min_path = paths(min_index,:);
disp(['Iteration ', num2str(iter), ': shortest path length = ', num2str(min_length)]);
disp(min_path);
end
```
这段代码中,我们使用了MATLAB内置的pdist2函数来计算城市之间的距离,使用了randsample函数来根据概率选择下一个城市,使用了sub2ind函数来计算路径长度。在每次迭代结束后,我们输出当前最短路径的长度和路径。
相关推荐
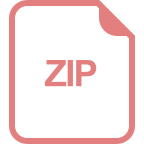
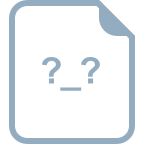
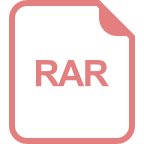














