在不适用opencv和imagemagick的基础上用C语言读入一张图像并对其进行中值滤波处理
时间: 2023-12-03 22:05:06 浏览: 28
如果不使用图像处理库,可以手动读取图像的像素值并对其进行中值滤波处理。以下是基于这种方法的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define STBI_ONLY_PNG
#define STB_IMAGE_IMPLEMENTATION
#include "stb_image.h" //stb_image.h是一个轻量级的图像解码库
#define STB_IMAGE_WRITE_IMPLEMENTATION
#include "stb_image_write.h" //stb_image_write.h是一个轻量级的图像编码库
//计算一维中值
int median(int* arr, int size) {
int i, j, temp;
for (i = 0; i < size - 1; i++) {
for (j = i + 1; j < size; j++) {
if (arr[i] > arr[j]) {
temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
}
if (size % 2 == 0) {
return (arr[size / 2 - 1] + arr[size / 2]) / 2;
}
else {
return arr[size / 2];
}
}
int main(int argc, char** argv)
{
if (argc != 3)
{
printf("Usage: %s <input_image_path> <output_image_path>\n", argv[0]);
return -1;
}
int width, height, channels;
unsigned char* image_data = stbi_load(argv[1], &width, &height, &channels, 0);
if (!image_data)
{
printf("Could not open or find the image: %s\n", argv[1]);
return -1;
}
unsigned char* image_data_filtered = (unsigned char*)malloc(width * height * channels * sizeof(unsigned char));
int window_size = 5;
int window_half_size = window_size / 2;
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
for (int c = 0; c < channels; c++) {
int values[25];
int count = 0;
for (int wy = -window_half_size; wy <= window_half_size; wy++) {
for (int wx = -window_half_size; wx <= window_half_size; wx++) {
int nx = x + wx;
int ny = y + wy;
if (nx >= 0 && nx < width && ny >= 0 && ny < height) {
values[count] = image_data[(ny * width + nx) * channels + c];
count++;
}
}
}
image_data_filtered[(y * width + x) * channels + c] = median(values, count);
}
}
}
stbi_write_png(argv[2], width, height, channels, image_data_filtered, width * channels);
stbi_image_free(image_data);
free(image_data_filtered);
return 0;
}
```
此代码中使用了stb_image.h和stb_image_write.h两个轻量级的图像处理库,其中stb_image.h用于读取图像,stb_image_write.h用于保存图像。中值滤波处理的实现使用了一个$5 \times 5$的窗口,对于每个像素的每个通道,先将窗口内的像素值存入一个一维数组中,然后对该数组进行排序并取其中值作为该像素通道的新值。
相关推荐
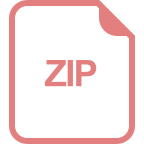
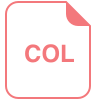
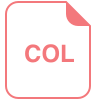
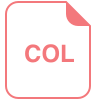
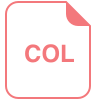
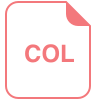









