代码解析 static int32_t CompareFileContent() { int32_t ret = -1; ifstream infile1, infile2; infile1.open("/data/test_aes.txt", ios::in|ios::binary); infile1.seekg (0, infile1.end); uint32_t length1 = infile1.tellg(); infile1.seekg (0, infile1.beg); infile2.open("/data/test_aes_new.txt", ios::in|ios::binary); infile2.seekg (0, infile2.end); uint32_t length2 = infile2.tellg(); infile2.seekg (0, infile2.beg); if (length1 != length2) { return ret; } uint8_t buffer1[FILE_BLOCK_SIZE] = {0}; uint8_t buffer2[FILE_BLOCK_SIZE] = {0}; for (uint32_t i = 0; i < length1 / FILE_BLOCK_SIZE; i++) { infile1.read(reinterpret_cast<char *>(buffer1), FILE_BLOCK_SIZE); infile2.read(reinterpret_cast<char *>(buffer2), FILE_BLOCK_SIZE); ret = memcmp(buffer1, buffer2, FILE_BLOCK_SIZE); if (ret != 0) { goto CLEAR_UP; } } CLEAR_UP: infile1.close(); infile2.close(); return ret; }
时间: 2024-04-03 09:37:24 浏览: 15
这段代码的作用是比较两个文件的内容是否相同。
首先,代码打开两个文件`/data/test_aes.txt`和`/data/test_aes_new.txt`,并获取它们的长度。
然后,代码比较两个文件的长度是否相同,如果长度不同,说明文件内容不同,返回`-1`。
接下来,代码定义两个缓冲区`buffer1`和`buffer2`,大小为`FILE_BLOCK_SIZE`,并循环读取两个文件的数据到这两个缓冲区中,再通过`memcmp`函数比较两个缓冲区的内容是否相同。如果不同,则说明文件内容不同,返回`-1`,否则继续循环。
最后,代码关闭两个文件句柄并返回比较结果。
相关问题
代码解析 static int32_t AesMultiBlockEncrypt(HcfCipher *cipher, HcfSymKey *key, HcfParamsSpec *params) { HcfBlob output = {}; ifstream infile; ofstream outfile; infile.open("/data/test_aes.txt", ios::in|ios::binary); infile.seekg (0, infile.end); uint32_t length = infile.tellg(); infile.seekg (0, infile.beg); uint8_t buffer[1024] = {0}; outfile.open("/data/test_aes_enc.txt", ios::out|ios::binary); HcfBlob input = {.data = (uint8_t *)buffer, .len = FILE_BLOCK_SIZE}; uint32_t count = length / FILE_BLOCK_SIZE; int32_t ret = cipher->init(cipher, ENCRYPT_MODE, (HcfKey *)key, params); if (ret != 0) { LOGE("init failed! %d", ret); goto CLEAR_UP; } for (uint32_t i = 0; i < count; i++) { infile.read(reinterpret_cast<char *>(buffer), FILE_BLOCK_SIZE); ret = cipher->update(cipher, &input, &output); if (ret != 0) { LOGE("update failed!"); goto CLEAR_UP; } if (output.data != nullptr && output.len > 0) { outfile.write(reinterpret_cast<const char *>(output.data), output.len); } if (output.data != nullptr) { HcfFree(output.data); output.data = nullptr; } } ret = cipher->doFinal(cipher, nullptr, &output); if (ret != 0) { LOGE("doFinal failed!"); goto CLEAR_UP; } if (output.data != nullptr && output.len > 0) { outfile.write((const char *)output.data, output.len); } if (output.data != nullptr) { HcfFree(output.data); output.data = nullptr; } CLEAR_UP: outfile.close(); infile.close(); return ret; }
这段代码使用了一个加密库(`cipher`)对一个文件进行多块加密,加密后将结果写入到另一个文件中。
首先,代码打开一个文件`/data/test_aes.txt`,获取它的长度,并定义一个大小为`1024`的缓冲区`buffer`。另外,代码打开一个输出文件`/data/test_aes_enc.txt`,以便将加密后的结果写入其中。
然后,代码根据加密算法、密钥和参数初始化加密库,并循环读取`count`个块的数据到缓冲区中,再将缓冲区的内容加密并输出到输出缓冲区`output`中。如果加密失败,代码会打印错误信息并跳转到`CLEAR_UP`标签处执行清除操作。
接下来,代码调用`doFinal`函数对最后一块数据进行加密,并将结果输出到输出缓冲区`output`中。如果加密失败,代码会打印错误信息并跳转到`CLEAR_UP`标签处执行清除操作。
最后,代码关闭输入和输出文件句柄并返回加密结果。
c++ zlib 解压 2MB的gzip字符串
对于2MB大小的gzip字符串,可以使用上面的代码进行解压,只需要将缓冲区的大小调整为2MB即可。修改代码如下:
```c++
#include <iostream>
#include <fstream>
#include <sstream>
#include <cstring>
#include <stdexcept>
#include <zlib.h>
std::string gzip_decompress(const std::string& compressed_string) {
const size_t BUFFER_SIZE = 2 * 1024 * 1024; // 修改为2MB
char buffer[BUFFER_SIZE];
std::stringstream uncompressed_stream;
z_stream zs;
memset(&zs, 0, sizeof(zs));
if (inflateInit2(&zs, 16 + MAX_WBITS) != Z_OK) {
throw std::runtime_error("inflateInit2 failed while decompressing.");
}
zs.next_in = (Bytef*)compressed_string.data();
zs.avail_in = compressed_string.size();
int ret;
do {
zs.next_out = reinterpret_cast<Bytef*>(buffer);
zs.avail_out = BUFFER_SIZE;
ret = inflate(&zs, 0);
if (uncompressed_stream.tellp() < 0) {
throw std::runtime_error("uncompressed stream error while decompressing.");
}
uncompressed_stream.write(buffer, BUFFER_SIZE - zs.avail_out);
} while (ret == Z_OK);
inflateEnd(&zs);
if (ret != Z_STREAM_END) {
throw std::runtime_error("corrupted compressed string while decompressing.");
}
return uncompressed_stream.str();
}
int main() {
std::ifstream input_file("compressed.gz", std::ios::in | std::ios::binary);
std::stringstream compressed_stream;
compressed_stream << input_file.rdbuf();
std::string compressed_string = compressed_stream.str();
std::string uncompressed_string = gzip_decompress(compressed_string);
std::cout << uncompressed_string << std::endl;
return 0;
}
```
在上面的代码中,我们将缓冲区的大小修改为2MB,这样就可以处理2MB大小的gzip字符串了。
相关推荐
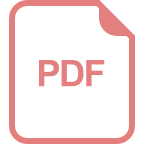
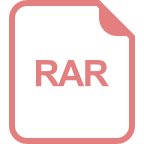








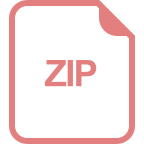
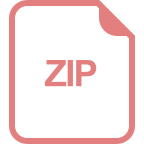
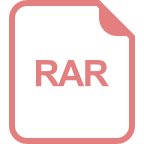