java实现五子棋游戏
时间: 2023-07-11 20:22:49 浏览: 113
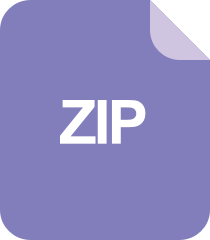
java实现五子棋
以下是 Java 实现五子棋游戏的简单步骤:
1. 创建一个棋盘类(Board),在棋盘上绘制出 15×15 的网格。
2. 定义一个棋子类(Piece),包括棋子的颜色和位置。
3. 创建一个游戏类(Game),它包括一个棋盘和两个玩家。可以通过键盘输入或鼠标点击来落子。
4. 实现落子规则,每当一个玩家下完一步棋后,判断是否有五个同色棋子相连,如果有则该玩家获胜。
5. 实现 AI 玩家,让计算机能够下棋。可以使用简单的算法,如随机下棋或简单的搜索算法。
以下是示例代码:
棋盘类 Board:
```
public class Board {
private int[][] grid;
public Board() {
grid = new int[15][15];
}
public void draw() {
// 绘制棋盘
}
public boolean isValidMove(int x, int y) {
// 判断落子是否合法
}
public void placePiece(Piece piece) {
// 在棋盘上放置棋子
}
public boolean checkWin(Piece piece) {
// 判断是否获胜
}
}
```
棋子类 Piece:
```
public class Piece {
private int x;
private int y;
private int color;
public Piece(int x, int y, int color) {
this.x = x;
this.y = y;
this.color = color;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public int getColor() {
return color;
}
}
```
游戏类 Game:
```
import java.util.Scanner;
public class Game {
private Board board;
private Player player1;
private Player player2;
private Player currentPlayer;
public Game() {
board = new Board();
player1 = new Player("Player 1", 1);
player2 = new AIPlayer("AI", 2);
currentPlayer = player1;
}
public void start() {
while (true) {
board.draw();
System.out.println(currentPlayer.getName() + " turn.");
int x, y;
if (currentPlayer instanceof AIPlayer) {
x = ((AIPlayer) currentPlayer).getNextMove(board);
y = ((AIPlayer) currentPlayer).getNextMove(board);
System.out.println("AI placed a piece at (" + x + ", " + y + ")");
} else {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter x: ");
x = scanner.nextInt();
System.out.print("Enter y: ");
y = scanner.nextInt();
}
Piece piece = new Piece(x, y, currentPlayer.getColor());
if (board.isValidMove(x, y)) {
board.placePiece(piece);
if (board.checkWin(piece)) {
System.out.println(currentPlayer.getName() + " wins!");
break;
}
currentPlayer = (currentPlayer == player1) ? player2 : player1;
} else {
System.out.println("Invalid move.");
}
}
}
}
```
玩家类 Player:
```
public class Player {
private String name;
private int color;
public Player(String name, int color) {
this.name = name;
this.color = color;
}
public String getName() {
return name;
}
public int getColor() {
return color;
}
}
```
AI 玩家类 AIPlayer:
```
import java.util.Random;
public class AIPlayer extends Player {
private Random random;
public AIPlayer(String name, int color) {
super(name, color);
random = new Random();
}
public int getNextMove(Board board) {
int x = random.nextInt(15);
int y = random.nextInt(15);
while (!board.isValidMove(x, y)) {
x = random.nextInt(15);
y = random.nextInt(15);
}
return x;
}
}
```
以上是一个基本的五子棋游戏实现,可以根据需求进行修改和扩展。
阅读全文
相关推荐
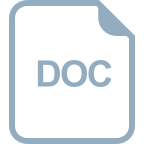
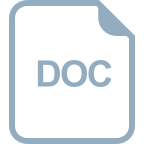
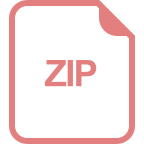
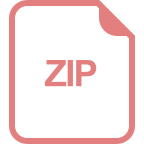
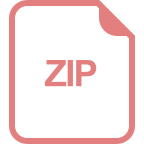
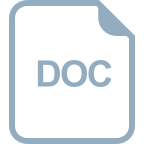
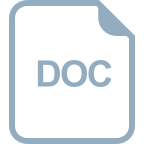
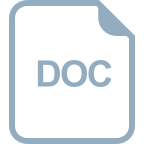
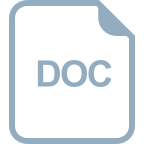
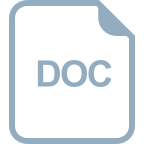
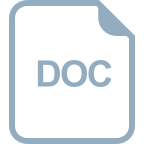
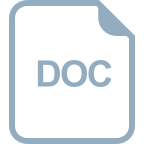
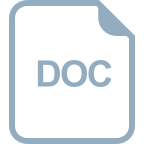
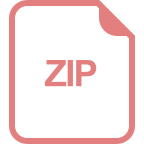